Introduction: Tools for NodeJS Development
Node.js has become a powerful runtime environment and using that we can build scalable and efficient applications. With Node.js, it’s possible to code both the front-end and back-end of a web application using JavaScript. This simplifies the process for full-stack developers of NodeJS Development Company, as they can create comprehensive web applications by mastering just JavaScript. Various tools for NodeJS development are available to improve productivity, debugging, and scaling applications in several ways:
Productivity Improvement
Package Management Tools like NPM (Node Package Manager) comes bundled with Node.js, is the default package manager. It manages dependencies, scripts and versioning. Alternative to NPM, YARN is another Package Management Tool with faster performance and better dependency management.
Code Editors and IDEs
IDE like Visual Studio Code (VS Code) is very lightweight and powerful with Node.js debugging support. It supports extensions for linting, formatting and auto completion along with built in terminal for running Node.js scripts. Besides this, another IDE named WebStorm is a feature rich IDE by JetBrains with excellent TypeScript support. It has built-in debugger and intelligent code assistance.
Debugging Tools for NodeJS Development
Node.js debugger (Node Inspect) is powerful debugging tool which helps developers to add breakpoints, find error full stack during errors to fix the same, thus help in maintaining good code quality. Chrome DevTools is another debugging tools for NodeJS development in Chrome, which can be attached to a running Node.js process using “chrome://inspect”. Another enhanced debugger is there called “ndb” with better breakpoints and async stack traces.
Linting and Formatting Tools
Linting tool enforces coding standards and best practices, which is most important while working in a collaborative environment to maintain code quality. Another tool like Prettier auto formats code for consistency, enhancing the developer readability.
Testing Frameworks
Frameworks like Jest with built-in assertions helps to write unit testing, integration testing as well as functional testing, which helps to maintain the stability of the application during CI/CD. Mocha & Chai is another flexible test framework, where Chai is an assertion library for BDD and TDD.
Performance Monitoring Tools
PM2 (Process Manager 2) manages and monitors NodeJS development performance in production. It can even detect memory leaks and has cluster mode feature to scale NodeJS applications.
Build and Automation Tools
Webpack can bundles JavaScript modules and assets making it production ready and reduces load times and optimizes performance. Gulp, another build tool, automates repetitive tasks like minification and compilation.
Top Tools for NodeJS Development in 2025
We will be discussing about following top tools for NodeJS development in details:
- Package Management Tools
- Scaffolding Tools
- Version Control
- Configuration Management
- Code Linting and Formatting tool
- Testing Tools
- Containerization and Deployment Tools
1) Package Management Tools for NodeJS
1.1 npm (Node Package Manager)
=> Default package manager for Node.js.
=> Manages dependencies, scripts and configurations.
=> Example:
- npm init y
- npm install express
1.2 Yarn
=> Alternative package manager with improved speed and security.
=> Example:
- yarn init y
- yarn add express
2) Project Scaffolding Tools for NodeJS
2.1 Yeoman
=> Generates project structures using predefined templates.
=> Example:
- npm install g yo
- yo node
2.2 Express Generator
=> Quickly scaffolds an Express.js application.
=> Example:
- npx express generator my app no view
- cd my app && npm install
2.3 NestJS CLI
=> Generates modular NestJS applications.
=> Example:
- npm install g @nestjs/cli
- nest new my nest app
3) Version Control and Repository Setup Tools for NodeJS
3.1 Git
=> Manages source code versioning and collaboration.
=> Example:
- git init
- git add.
- git commit m “Initial commit”
3.2 Husky & Lint Staged
=> Adds pre commit hooks for enforcing code quality.
=> Example:
- npm install save dev husky lint staged
Add to `package.json`:
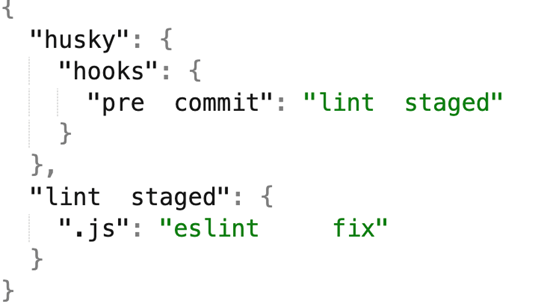
4) Environment and Configuration Management Tools for NodeJS
4.1 dotenv
=> Manages environment variables.
=> Example:
- npm install dotenv
=> Create `.env` file:
Env PORT=3000
=> Use in `index.js`:
- require(‘dotenv’).config();
- console.log(process.env.PORT);
4.2 Config
=> Hand les multi environment configuration management.
=> Example:
- npm install config
=> Create `config/default.json`:
{
"port": 3000
}
=> Use in `server.js`:
- const config = require(‘config’);
- console.log(config.get(‘port’));
5) Code Linting and Formatting Tools for NodeJS
5.1 ESLint
=> Ensures consistent code style and best practices.
=> Example:
- npm install save dev eslint
- eslint init
5.2 Prettier
=> Auto formats code for readability.
=> Example:
- npm install save dev prettier
- prettier write .
6) Testing Frameworks Tools for NodeJS
6.1 Jest
=> Popular testing framework with built in assertions.
=> Example:
- npm install save dev jest
=> Create `sum.js`:
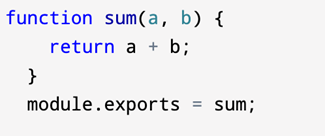
=> Create `sum.test.js`:
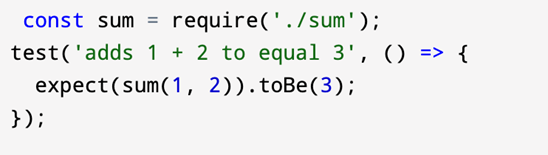
=> Run tests:
- jest
7) Containerization and Deployment Tools for NodeJS
7.1 Docker
=> Containers Node.js applications for consistent deployment.
=> Example:
- dockerfile
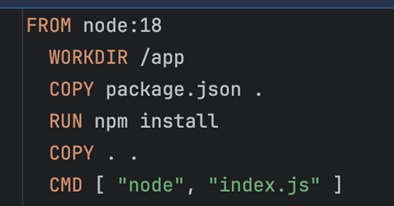
7.2 Kubernetes
=> Orchestrates Node.js applications at scale.
=> Example:
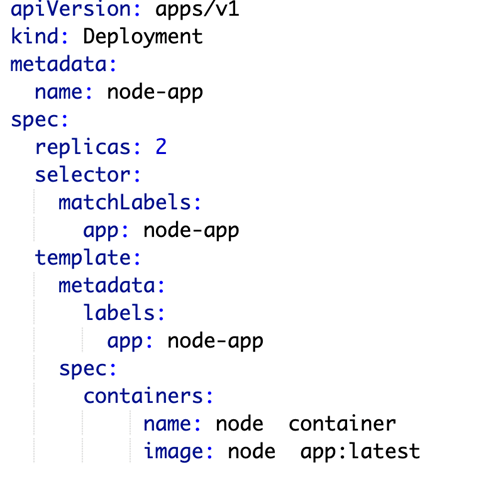
Debugging Tools for NodeJS
Debugging is not a simple thing, and it is essential aspect of Node.js development. Whether you work on a stand-alone application or a complex microservices architecture, I have explained in depth about debugging tools for NodeJS. It covers logging, profiling and remote debugging techniques.
1) Debugging Stand-alone Node.js Applications
1.1 Node.js Built in Debugger
=> Comes with Node.js and allows debugging directly from the command line.
=> Example:
- nodes inspect app.js
=> Use breakpoints:
debugger
- console.log (“Debugging Node.js”);
=> Debug with Chrome DevTools:
- node inspect brk app.js
1.2 Chrome DevTools
=> Open `chrome://inspect` in Google Chrome.
=> Click on the `Remote Target` section to inspect the running Node.js instance.
=> Provides features like breakpoints, step execution and real time variable inspection.
1.3 Visual Studio Code Debugging
=> VS Code supports direct debugging with `launch.json`.
=> Example configuration:
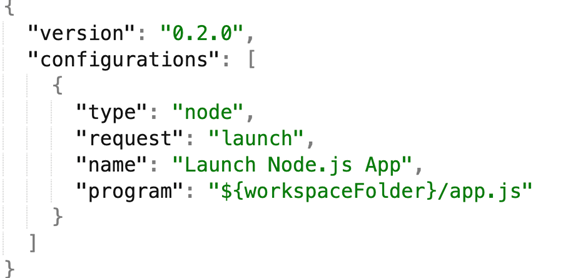
=> Start debugging using the Run and Debug panel.
1.4 Winston for Logging
=> Winston is a popular logging library for structured logs.
=> Example:
- npm install winston
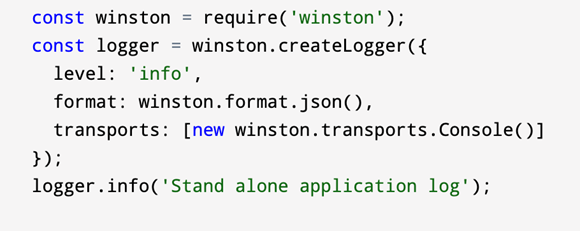
1.5 Node.js Performance Hooks
=> Measures execution time for different parts of the application.
=> Example:
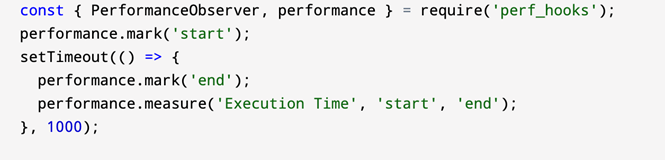
2) Debugging Microservices in Node.js
2.1 Distributed Logging with Pino
=> Pino is optimized for high speed logging in microservices.
=> Example:
- npm install pino
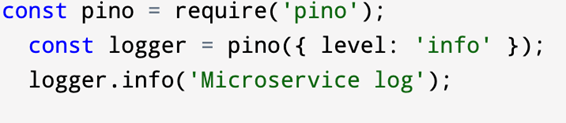
2.2 OpenTelemetry for Tracing
=> Enables distributed tracing across microservices.
=> Example:
- npm install @opentelemetry/api @opentelemetry/node

2.3 Jaeger for Distributed Tracing
=> Helps analyze requests flowing through microservices.
=> Example:
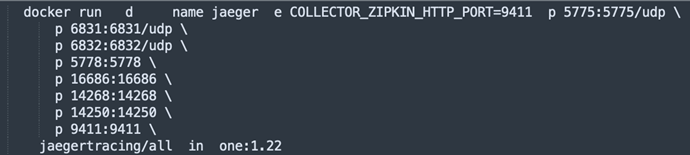
=> Integrate with Node.js:

2.4 Debugging with PM2
=> Process manager for Node.js applications in production.
=> Example:
- npm install g pm2
- pm2 start app.js watch
- pm2 logs
2.5 Kubernetes Debugging with Kubectl
=> Inspect running microservices on Kubernetes.
=> Example:
- kubectl logs f my node service
- Access ell inside a pod:
- kubectl exec it my node service /bin/
2.6 Debugging HTTP Requests with Postman & cURL
=> Postman provides a UI to test microservices endpoints.
=> Example cURL command:
- curl X GET http://localhost:3000/api/orders
2.7 Remote Debugging with VS Code
=> Debug a microservice running in a container.
=> Example `launch.json`:
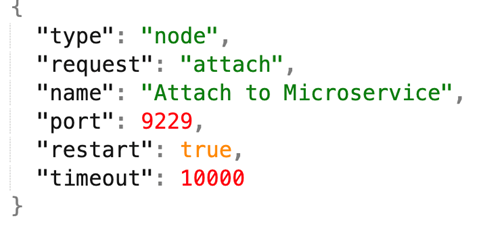
=> Start Node.js with:
- node inspect=0.0.0.0:9229 app.js
Testing Tools for NodeJS
Testing tools for NodeJS ensures reliability, performance and security. Your Node.js applications be it stand alone or microservices based but it requires different testing strategies.
1) Testing Stand-alone Node.js Applications
1.1 Unit Testing with Jest
=> Jest is a popular testing framework with built in assertions, mocks and snapot testing.
=> Install Jest:
- npm install save dev jest
=> Example test case:
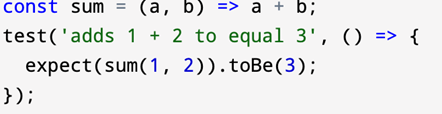
=> Run tests:
- npx jest
1.2 Mocha and Chai for BDD/TDD
=> Mocha is a flexible test framework, while Chai provides assertion capabilities.
=> Install:
- npm install save dev mocha chai
=> Example test:
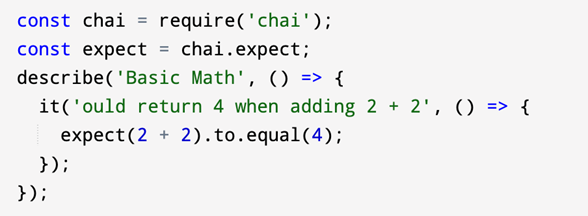
=> Run tests:
- npx mocha
1.3 Supertest for API Testing
=> Supertest simplifies HTTP request assertions.
=> Install:
- npm install save dev supertest
=> Example:
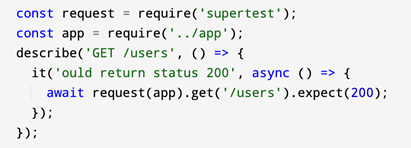
1.4 Load Testing with Autocannon
=> Simulates concurrent users.
=> Install:
- npm install g autocannon
=> Example:
- autocannon c 100 d 10 http://localhost:3000
1.5 Static Analysis with ESLint
=> Detects code issues early.
=> Install:
- npm install save dev eslint
=> Initialize and run:
- npx eslint.
2) Testing Node.js Microservices
2.1 Contract Testing with Pact
=> Ensures service contracts are upheld.
=> Install:
npm install save dev @pact foundation/pact
=> Example:

2.2 Integration Testing with Postman & Newman
=> Postman provides an API test UI and Newman runs them via CLI.
=> Install:
- npm install g newman
=> Run tests:
- newman run api_tests.postman_collection.json
2.3 Load Testing with K6
=> Simulates API stress.
=> Install:
npm install g k6
=> Example test script `test.js`:
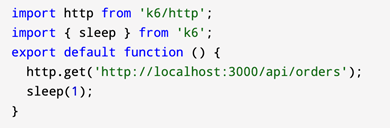
=> Run:
- k6 run test.js
2.4 Security Testing with OWASP ZAP
=> Scans for vulnerabilities.
=> Install and run:

2.5 Distributed Testing with Cypress
=> Automates UI and API tests.
=> Install:
- npm install save dev cypress
=> Example test:
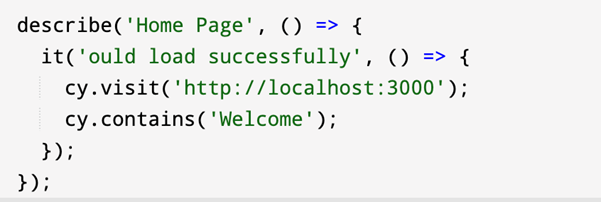
2.6 Kubernetes Testing with Kubectl & Helm
=> Ensure deployed microservices are functional.
=> Example test:
- kubectl get pods
- kubectl logs my node service
2.7 Chaos Testing with Gremlin
=> Tests resilience by simulating failures.
=> Example:
- gremlin attack container network latency target node app
Package Management Tools for NodeJS
Package management also one of the important tools for NodeJS as it enables developers to install, update and manage dependencies efficiently. Node.js offers multiple package management tools and each has its unique capabilities that enhance performance, security and ease of use.
1) Understanding Package Managers in Node.js
Package managers handle the installation and dependency management of JS libraries and frameworks. There are three primary package managers in the Node.js and these are as bellows:
- npm (Node Package Manager) – The default package manager for Node.js.
- Yarn – An alternative package manager focused on speed and consistency.
- pnpm – A modern package manager with optimized disk usage and performance.
2) npm: The Default Package Manager
2.1 Installing and Managing Packages with npm
=> Install a package globally:
- npm install g typescript
- To install a package locally: npm install express
- To to Install dependencies from `package.json`: npm install
2.2 Package Versioning in npm
=> Specify a fixed version:
“express”: “4.18.2”
=> Allow minor updates (`^`):
“express”: “^4.18.0”
=> Allow patch updates (`~`):
“express”: “~4.18.2”
2.3 npm Workspaces for Monorepos
=> Configure workspaces in `package.json`:
“workspaces”: [“packages/”]
=> To install dependencies for all workspaces: npm install
2.4 Security Auditing with npm
=> Run a security audit:
- npm audit
=> Fix vulnerabilities automatically:
- npm audit fix
2.5 Performance Optimizations in npm
=> Use the cache for faster installations:
- npm cache verify
=> To install dependencies using CI friendly mode:
- npm ci
3) Yarn: Faster and More Reliable Package Management
3.1 Installing and Using Yarn
=> Install Yarn:
- npm install g yarn
=> To install a package:
- yarn add express
=> Remove a package:
- yarn remove express
3.2 Workspaces in Yarn
=> Define workspaces in `package.json`:
- “workspaces”: [“apps/”]
=> To install all workspace dependencies: yarn install
3.3 Deterministic Dependency Resolution with Yarn
=> Lock dependencies using `yarn.lock`:
- yarn install frozen lockfile
3.4 Yarn Plug’n’Play (PnP) for Faster Dependency Resolution
=> Enable PnP in `.yarnrc.yml`:
- nodeLinker: “pnp”
3.5 Performance Enhancements in Yarn
=> Run parallel installations:
- yarn install network timeout 1000000
- Enable caching for offline use: yarn config set enableGlobalCache true
4) pnpm: The Modern and Efficient Package Manager
4.1 Installing and Using pnpm
=> Install pnpm globally:
- npm install g pnpm
=> To Install dependencies using pnpm: pnpm install
4.2 Performance Advantages of pnpm
=> Uses a global store instead of duplicating dependencies.
=> Installs packages symlinked to a global store, reducing disk usage.
4.3 Workspaces with pnpm
=> Enable workspaces in `pnpm workspace.yaml`:
- packages: “apps/”
=> Install all workspace dependencies: pnpm install
4.4 Strict Dependency Resolution in pnpm
=> Enforce strict dependencies:
pnpm install strict peer dependencies
4.5 Speed Optimization with pnpm
=> Enable fast installations:
- pnpm install frozen lockfile
=> Perform a deep clean of dependencies: pnpm prune
How to use TypeScript with Node.js effectively?
TypeScript has become the standard for modern Node.js development as it offers type safety and better tooling, and it enhances developer productivity. So to integrate TypeScript requires the right set of tools for NodeJS to streamline compilation, linting, testing and deployment.
1) Setting Up TypeScript in a Node.js Project
1.1 Installing TypeScript
=> To begin using TypeScript, install it globally or locally in your Node.js project:
- npm install g typescript
- npm install save dev typescript
1.2 Initializing TypeScript Configuration
=> Generate a `tsconfig.json` file using:
tsconfig.json init
=> A basic `tsconfig.json`:
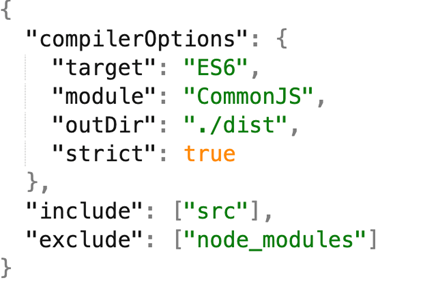
2) TypeScript Compilation and Build Tools for NodeJS
2.1 `tsc`: TypeScript Compiler
=> TypeScript’s built in compiler converts `.ts` files into JavaScript:
- tsс build
=> For an optimized build, enable `incremental` mode in `tsconfig.json`:
- “incremental”: true,
2.2 ts node: Execute TypeScript Without Compiling
=> `ts node` allows running TypeScript files directly:
- npm install save dev ts node
=> Run a script:
- ts node src/index.ts
2.3 Webpack with TypeScript
=> For bundling TypeScript projects:
- npm install save dev webpack webpack cli ts loader
=> Example `webpack.config.js`:
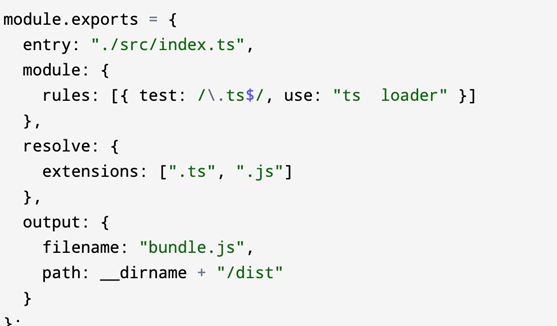
Build using:
- npx webpack
3) Linting and Code Quality Tools for NodeJS
3.1 ESLint with TypeScript
=> Install ESLint and TypeScript support:
npm install save dev eslint @typescript eslint/parser @typescript eslint/eslint plugin
=> Configure `. eslintrc.js`:
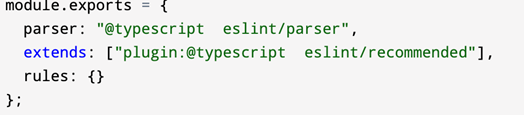
=> Run ESLint: npx eslint src//.ts
3.2 Prettier for Code Formatting
=> Install Prettier:
- npm install save dev prettier
=> Format TypeScript files:
- npx prettier write src//.ts
4) Testing TypeScript in Node.js
4.1 Jest for Unit Testing
=> Install Jest with TypeScript support:
- npm install save dev jest ts jest @types/jest
=> Configure `jest.config.js`:
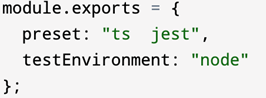
=> Run tests: npx jest
4.2 Mocha & Chai for BDD Testing
=> To Install dependencies:
- npm install save dev mocha chai @types/mocha @types/chai ts node
=> Run tests:
- npx mocha r ts node/register “src//.test.ts”
5) TypeScript Integration with Node.js Frameworks
5.1 Express with TypeScript
=> Install Express and types:
- npm install express @types/express
=> Example: Express server in TypeScript
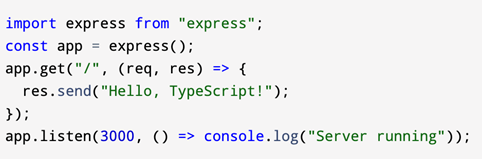
=> Compile and run:
ts node src/server.ts
5.2 NestJS: Scalable TypeScript Framework
=> NestJS is a fully TypeScript native framework:
- npm install g @nestjs/cli
- nest new my nest app
=> Example controller:
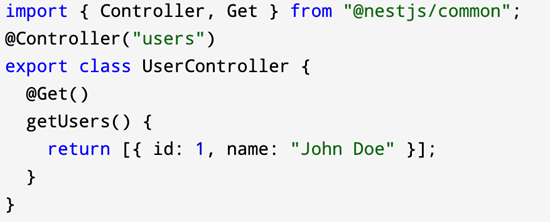
=> Run the app:
- npm run start
6) Type Checking and Static Analysis
6.1 Type Checking with `tsc noEmit`
=> Run type checks without compiling: tsс noEmit
6.2 TypeDoc for Documentation
=> Generate documentation:
- npm install save dev typedoc
- npx typedoc out docs src
Security Tools for NodeJS Development
In current development environments, microservices architectures is the go to pattern for large scale distributed applications. And microservices are widely adopted because of their scalability, flexibility and resilience characteristics. Here, I have explained how to implement complex security mechanisms in a Node.js based microservices architecture.
1) Nature of Microservices Security
=> Microservices are often interconnected through APIs, which makes security critical at multiple levels:
- Service to Service Authentication: It ensures that communication between services is secure & trusted.
- API Security: Need to protect the API endpoints exposed by each service.
- Data Integrity and Privacy: We need to encrypt sensitive data to ensure data integrity.
- Real-Time Monitoring and Logging: Keeping track of activities across services for anomaly detection and compliance.
=> Given these challenges, a combination of techniques and tools for NodeJS is required to ensure the security of a microservices based application.
2) Core Security Tools and Techniques
2.1 Authentication and Authorization with JWT
=> In microservices, JSON Web Tokens (JWT) are frequently used for authentication & authorization.
=> JWT allows services to securely communicate and verify the identity of users and other services.
=> Example: JWT Authentication in Node.js
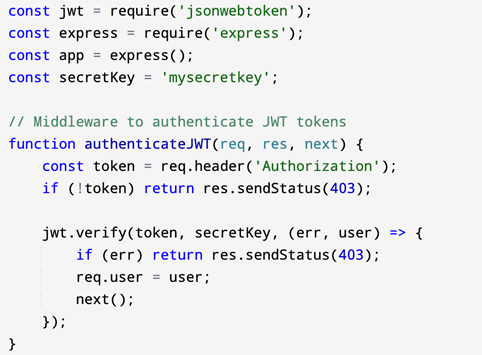
/A route to issue a JWT
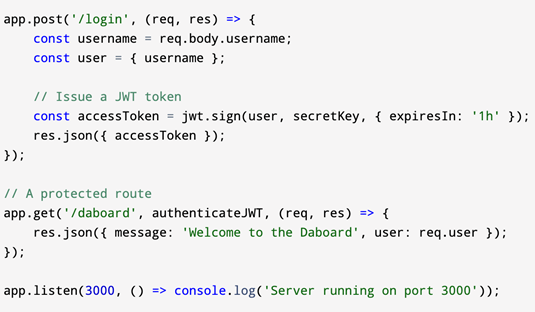
=> In this example:
- The `/login` route generates a JWT token after validating the user credentials.
- The `/daboard` route is protected by the `authenticateJWT` middleware, which ensures that the user is authorized to access the resource.
2.2 API Gateway Security with Rate Limiting
=> An API Gateway serves as the entry point for all microservices and It also helps in securing the system. If we implement rate limiting, then it can help prevent abuse and mitigate DDoS attacks.
=> Example: Implementing Rate Limiting with `express rate limit`
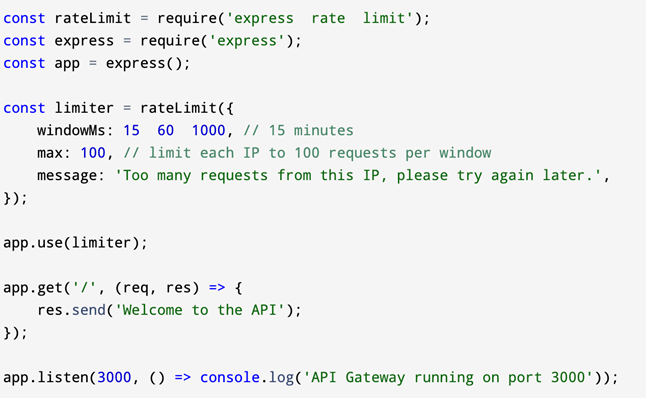
2.3 Input Validation with `Joi`
=> In any application, improper input validation is a common source of vulnerabilities like SQL injection and XSS. To secure microservices, you need to validate and sanitize user input before processing it.
=> Example: Input Validation with `Joi`
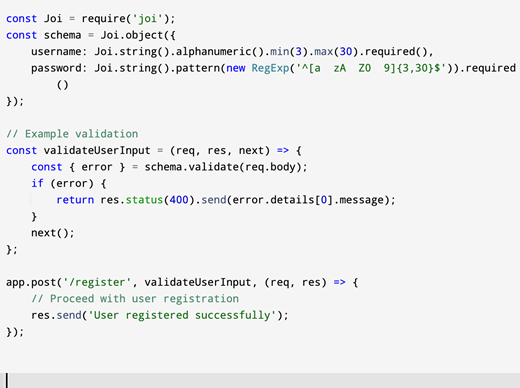
=> In this code:
- Joi is used to define a schema that checks the format and requirements of `username` and `password` fields.
- If the input doesn’t match the schema, a 400 Bad Request response is returned.
2.4 Encryption for Sensitive Data
=> Microservices often handle sensitive data like personal information and payment details. It is crucial to encrypt this data both at rest and in transit to avoid breaches.
=> Example: Encrypting Data with `crypto`
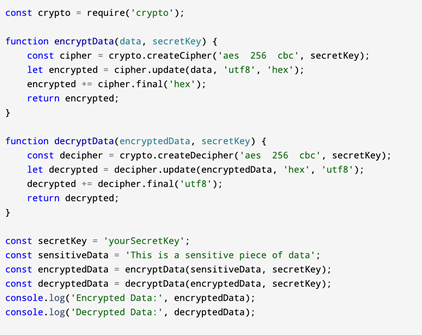
2.5 Real Time Monitoring with Prometheus and Grafana
=> Real time monitoring allows you to track security incidents and service health. By integrating Prometheus and Grafana with your microservices, you can collect metrics and visualize the performance of your services.
=> Example: Exposing Prometheus Metrics
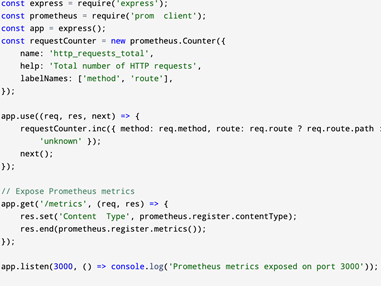
=> In this code:
- We create a counter metric to track the number of HTTP requests.
- Prometheus can scrape the `/metrics` endpoint to collect these metrics and Grafana can be used to visualize them in real time dashboards.
Best IDEs for Node.js Development
Below are most popular and effective IDEs and code editors for Node.js development.
- Visual Studio Code (VS Code)
- WebStorm
- Sublime
- Atom
1. Visual Studio Code (VS Code)
=> Key Features:
1) VS Code provides IntelliSense, which offers autocompletion, parameter hints and function signatures. It can also provide code suggestions based on the context, and this makes it easier to write JavaScript/Node.js code.
2) VS Code has built in debugging capabilities. You can easily set breakpoints, step through code, inspect variables and even run the code in the terminal.
3) VS Code has a rich ecosystem of extensions. Popular Node.js related extensions include:
- ESLint: A linter to maintain code quality.
- Code formatting.
=> Pricing:
VS is free, and no cost involved here if you want to use
=> Node.js Modules Intellisense:
Autocompletion for Node.js built in modules.
=> Debugger for Chrome:
One of the useful tools for NodeJS application debugging with the Chrome browser.
=> Git Integration:
VS Code integrates directly with Git, allowing you to commit, pu and pull code right from the editor.
=> Why Choose VS Code for Node.js Development:
- Lightweight and fast.
- Large selection of extensions.
- Customizable for various workflows.
- Active community and continuous updates.
=> Example:
To set up a Node.js project in VS Code:
1. Install the Extension Pack for specific libraries and tools for NodeJS.
2. Configure debugging by creating a launch configuration (`launch.json`) to start the app and set breakpoints.
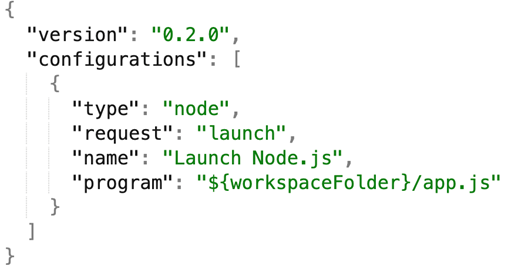
2. WebStorm
WebStorm is developed by JetBrains and is a premium IDE designed specifically for JavaScript and Node.js development and WebStorm is not open source, but it provides advanced features.
=> Key Features:
- WebStorm provides smart autocompletion for JavaScript, Node.js and many third-party libraries.
- Like VS Code, WebStorm offers an integrated debugger with visual tools. It works seamlessly with Node.js to help you debug and inspect applications.
- WebStorm offers advanced refactoring capabilities that includes renaming variables and functions and extracting method
- Native Git support enables you to easily manage code, branches and merges within the IDE.
- WebStorm offers integrated support for testing frameworks like Mocha, Jest and Jasmine.
- You can debug your Node.js code directly in the IDE without requiring additional setup.
=> Pricing:
This does not come for free, and price is according to subscription based like first yr, you have to pay approx. 60 $ for individual and 130$ for corporate.
=> Node.js Debugging
WebStorm offers out of the box support for Node.js debugging:
- Set breakpoints in your Node.js code.
- Run the app in Debug mode using the built-in tools.
- Inspect variables, step through functions and monitor console outputs in real time.
3. Sublime Text
Sublime Text is also popular & it focuses on speed, ease of use and extensibility. But it is not as feature rich as VS Code or WebStorm, but it is still preferable for minimalistic environments.
=> Key Features:
Sublime is known for its speed and low memory consumption.
We can install packages from the Package Control manager to enhance the editor’s capabilities. Some useful packages for Node.js development include:
- SublimeLinter: For linting Node.js code.
- Nodejs: For Node.js syntax highlighting and autocompletion.
- GitGutter: To see Git diff information directly in the editor.
- Multiple Cursors: A powerful feature for editing multiple lines of code at once.
=> Pricing:
This does not come for free, and price is according to subscription based like first yr, you have to pay approx. 100 $ for individual and 70$ / per user upto 10 uses can use for corporate.
=> To install Node.js related plugins in Sublime Text:
- Open the Command Palette (`Ctrl+ift+P` or `Cmd+ift+P`).
- Search for `Install Package` and press enter.
- Install packages like `Nodejs` for syntax highlighting or `SublimeLinter` for linting.
4. Atom
Atom is from GitHub, and it is open source text editor that is highly customizable and suitable for Node.js development.
=> Key Features:
Atom supports a vast library of packages for various languages, including Node.js. Some key packages include:
- Provides IDE like features for Node.js development.
- Integrates ESLint for JavaScript linting.
- Auto formatting for clean code.
- Atom offers built in GitHub integration and hence makes it easy to clone repositories, commit changes and updates.
- With custom themes, keybindings and snippets, Atom allows you to tailor the editor to your workflow.
- Atom supports cross platform as it runs on Windows, Mac and Linux.
=> Pricing:
This also comes without any cost
=> Example:
To start Node.js development in Atom:
- Install the ide node package to enable autocompletion, linting and debugging.
- Customize your workflow by adding GitHub integration or specific snippets for faster coding.
Node.js Frameworks
Here I have explained about some of the most popular and powerful Node.js frameworks that are ideal for developing complex applications and microservices. These frameworks also provide built-in tools for NodeJS and best practices for handling tasks such as routing, middleware management, data persistence, security and more.
Overview of Frameworks like Express.js, NestJS, Koa.js, and Hapi.js for building scalable applications.
1. Express.js
Express.js is the most widely used Node.js framework & it is a great fit for small to medium scale applications. It also scales well for more complex applications, especially when used with other libraries and tools for NodeJS.
=> Key Features:
- Express provides a simple and extensible routing mechanism that makes it easy to define URL paths and HTTP methods for each endpoint.
- It also supports middleware functions, allowing you to add functionality to the request response cycle such as logging, error handling and authentication.
- Express supports various template engines like EJS, Pug and Handle bars for rendering dynamic content.
- Integration with Databases: Express can be easily integrated with both SQL (For ex: MySQL, PostgreSQL) and NoSQL (e.g., MongoDB, Redis) databases.
=> When to Use Express.js:
- Ideal for developers who need a simple framework with minimal overhead.
- Great for building complex applications that require a custom structure and logic, as it doesn’t impose many restrictions.
=> Example:
Express.js Setup for a Basic API
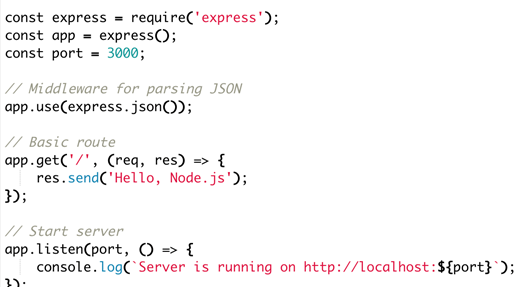
2. NestJS
NestJS built with TypeScript, and it is a modern & opinionated framework, and it is heavily inspired by Angular. It is mainly designed for building scalable and maintainable server-side applications and is perfect for complex enterprise level applications.
=> Key Features:
- NestJS encourages the use of modules, making it easy to organize the application into smaller, manageable pieces. Each module encapsulates related features such as controllers, services and providers.
- Like Angular, NestJS provides a powerful dependency injection system that simplifies the management of services and hence loose coupling is achieved between components.
- NestJS is built from the ground up with TypeScript that provides strong typing & better autocompletion and thereby it improves developer productivity. It includes built in support for building microservices with libraries like RabbitMQ, Kafka and gRPC.
=> When to Use NestJS:
- Ideal for large scale applications that require structure and scalability.
- Microservices: It’s perfect for building distributed systems and microservices architecture due to its out of the box support for microservices.
- If you prefer TypeScript over JavaScript, NestJS will leverage TypeScript full potential, providing a better development experience.
=> Example:
1) Install NestJS CLI
- npm install g @nestjs/cli
2) Create a new project
- nestjs new project name
3) Navigate into the project directory
- cd project name
4) Start the server
- npm run start
This will create a basic application where you can start adding your modules n controllers and services, structured neatly into directories.
3. Koa.js
Koa.js is another lightweight web framework, developed by Express.js creator. Unlike Express, Koa does not bundle middleware hence it gives developers more control over which modules they want to include in their applications.
=> Key Features:
- Koa is minimalistic in nature, and it allows you to build a fully custom framework based on your application’s needs.
- Koa natively supports async/await syntax that simplifies working with asynchronous code especially with database calls or external APIs.
- It uses a more powerful and flexible middleware system, enabling more control over how requests are handled.
- Koa has improved error hand ling capabilities, and it allows you to capture errors at any point in the middleware pipeline.
=> When to Use Koa.js:
- Perfect if you need more control over the stack and want to tailor the framework to your application’s needs.
- Ideal for applications that require clean, easy to maintain asynchronous code.
- While scalable, Koa is generally used for projects that require more custom hand ling, not necessarily for enterprise applications.
=> Example:
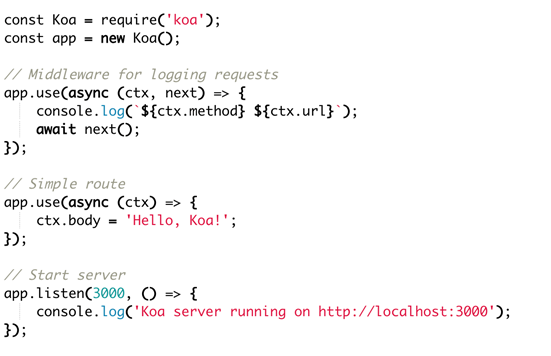
4. Hapi.js
Hapi.js is the goto framework for building web applications and APIs. Hapi is used for building complex applications that require intricate routing & detailed validations
=> Key Features:
- Hapi’s routing system is highly flexible, and it allows you to define detailed and customized routes based on HTTP methods, parameters and headers.
- Hapi includes a powerful validation system built on Joi so you can validate incoming requests easily.
- The Hapi ecosystem is built around plugins, so it is easy to extend the framework’s capabilities without modifying core code.
- Hapi comes with built in features like input validation, cross site request forgery (CSRF) protection and more.
=> When to Use Hapi.js:
- Hapi is a strong choice for building RESTful APIs with complex routing & validation
- Hapi is well suited for enterprise level applications that require a large number of features and detailed configuration.
=> Example: Hapi.js Setup
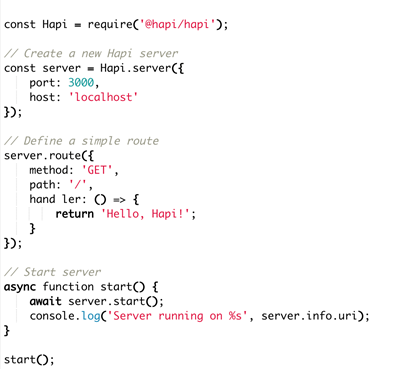
Advantages of Using TypeScript with Node.js
1. Static Typing:
- TypeScript provides static type checking at compile time. This helps catch errors early in the development process (before runtime) and hence it is useful in large, complex projects.
- With static typing, TypeScript enables you to define types for variables, function arguments, return values and even third-party libraries (via DefinitelyTyped or custom types).
2. Enhanced Developer Productivity:
- IDEs like VSCode provide intelligent code completion, tooltips and inline documentation when working with TypeScript, significantly improving developer experience.
- With TypeScript, navigating large codebases becomes easier. You can jump to function definitions, see type annotations and trace code paths more effectively.
- With TypeScript code refactoring is safer and easier because the compiler ensures that any changes you make do not break the application unexpectedly.
3. Improved Maintainability:
- In larger teams, static types make it easier for different developers to understand how data flows through the application and how various parts of the system are interconnected.
- Type annotations can also serve as documentation, making the codebase more understand able to others and even to future you!
4. Better Tooling and Ecosystem:
- Many modern Node.js frameworks and libraries, such as NestJS, TypeORM and Apollo Server, provide first class TypeScript support. These tools for NodeJS often leverage TypeScript type system to offer better developer experiences (e.g., auto completion, type safe APIs).
- TypeScript is a superset of JavaScript what does this mean? That all valid JavaScript code is valid TypeScript. You can incrementally adopt TypeScript in a Node.js project by gradually migrating files from JavaScript to TypeScript.
5. Scalability:
- For smaller projects, plain JavaScript can work just fine, but as the project scales, having types to track state and data flow can prevent bugs and help developers maintain large systems.
Example: TypeScript in a Node.js Project
Here’s how TypeScript might look in a basic Node.js project:
1. Install TypeScript and Dependencies
- npm install typescript @types/node save dev
2. Configure TypeScript
In the project root, create a `tsconfig.json` file:
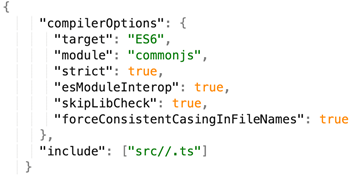
3. TypeScript Code Example
server.ts:
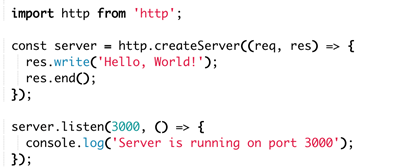
4. Compile and Run
To compile the TypeScript code, run: npx tsc
Then, to run the application: node dist/server.js
Node.js Build Tools
In this blog, I have mentioned most essential build and deployment tools for Node.JS design patterns that can help streamline the development process for complex applications. Automate tasks and bundle assets with Grunt, Webpack, and Gulp.
1. Grunt
Grunt is actually JS task runner, and it can automate your repetitive tasks for ex-minification, compilation and linting.
Install Grunt and Required Plugins:

Grunt can automate JavaScript minification.
=> GruntJS File:
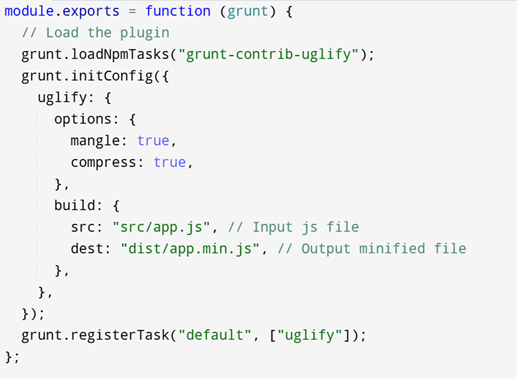
=> App.js src code
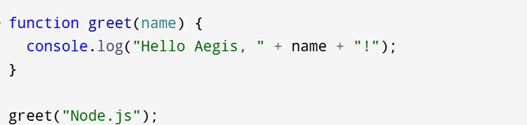
Then run : npx grunt
verify o/p: function greet(o){console.log(“Hello Aegis, “+o+”!”)}greet(“Node.js”);
2. Webpack
Webpack as a module bundler is mainly used for JavaScript applications. It bundles your source code into a single file/ a few files that can be easily loaded by the browser.
=> Key Features:
- Webpack allows you to bundle all your application files that includes JavaScript, CSS and assets like images and fonts, into optimized files.
- Webpack supports code splitting and it allows you to split your bundle into smaller pieces to improve the loading time of your application.
- It provides a wide array of loaders and plugins to transform and optimize source code for ex: transpiling ES6 to ES5 using Babel.
- During development, Webpack allows you to apply code changes instantly without reloading the entire page.
=> When to Use Webpack:
- In Complex Applications
- Code Optimization and better performance.
=> Example: Webpack Basic Configuration
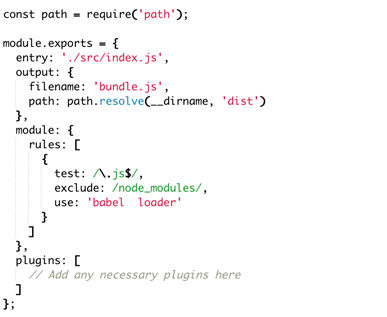
3. Gulp
=> Automates repetitive tasks like minification and transpiration.
=> Example:
- npm install save dev gulp
=> Create `gulpfile.js`:
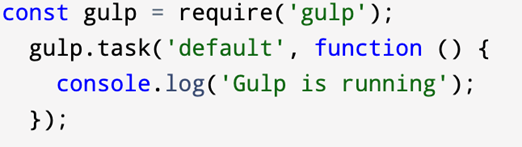
=> Run:
- gulp
npm (Node Package Manager)
npm can manage dependencies, scripts and modules within your Node.js application & it is the default package manager for node.
=> Key Features:
- npm handles both direct and development dependencies. It helps in keeping track of packages that your application depends on.
- npm allows you to define custom scripts to automate tasks for build test and deploy process
- It supports versioning of dependencies
=> Example: Common npm Scripts for Build and Deployment
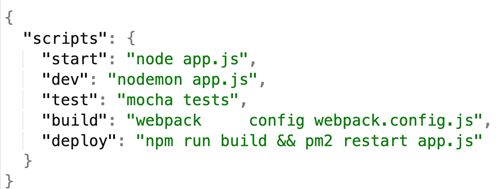
=> When to Use npm:
- Basic Package Management.
- Task Automation of your repetitive tasks.
Comparison of Popular Tools for NodeJS Development
1. NPM vs YARN
Npm and Yarn are interchangeable but they were both created to do one thing -> manage JavaScript dependencies in a Node. js project. There are performance level and workflow differences that may make one or the other preferable in certain cases. Here is a comparison of their similarities and differences:
=> Purpose and Similarity
- Npm (Node Package Manager) and Yarn (a native Facebook package manager) are both tools used to manage dependencies. They enable you to install and update third party libraries and packages in your project.
- npm is the default package manager and it comes with Node.js and this has been around since 2010.
- Yarn actually introduced in 2016 by Facebook, and it can address some of the performance and consistency issues that npm had at the time.
=> Package Installation
Both npm and Yarn can be used to to Install dependencies listed in a project’s `package.json`.
npm:
npm install
Yarn:
yarn install
- Both command s will install the dependencies defined in your `package.json`. If a `yarn.lock` file exists, Yarn will respect it and to Install dependencies accordingly. Similarly, npm now uses a `package lock.json` file which functions similarly to Yarn’s lock file.
=> Performance
- Yarn was originally created to solve performance issues with npm by introducing parallel installation of packages and caching. Yarn’s caching mechanism makes it faster in some scenarios, as it avoids downloading the same package multiple times.
- npm: In earlier versions, npm suffered from slow installs due to linear dependency resolution and lack of aggressive caching.
- Yarn: Introduced parallel installation, better caching and more efficient hand ling of dependencies, making it faster in many cases.
- However, npm has improved significantly in recent versions (starting from v5 and later), incorporating many features that made Yarn popular, such as:
- npm v5+: Improved performance and caching.
- npm v7+: Introduced support for workspaces, which was previously a feature of Yarn.
=> Lockfiles
- Both npm and Yarn use lock files to ensure consistent installations across environments, preventing the “works on my machine” problem.
- npm uses `package lock.json`, which ensures that everyone installs exactly the same version of each dependency.
- Yarn uses `yarn.lock`, which serves the same purpose as `package lock.json`.
Both lock files perform the same function and are not interchangeable—if you use npm, it will generate `package lock.json` and if you use Yarn, it will generate `yarn.lock`.
=> Workspaces
Yarn introduced the concept of workspaces to manage multiple packages in a single repository (monorepos). npm adopted workspaces in version 7, so both package managers now have similar support for managing multi package repositories.
- Yarn Workspaces: Allows you to manage dependencies across multiple packages in a monorepo.
- npm Workspaces (v7+): Provides similar functionality to Yarn workspaces.
=> Dependency Resolution
Both npm and Yarn resolve dependencies based on the `package.json` file, but their mechanisms differ slightly:
- npm: Uses a flat dependency structure in the `node_modules` folder, which means packages with the same version get installed only once at the top level.
- Yarn: Uses a deterministic resolution strategy, where dependencies are locked into specific versions to ensure the same results across all installations.
Both managers now handle dependency resolution in a fairly similar way, but Yarn was originally seen as being more predictable in dependency resolution due to its lock file.
=> CLI Command Differences
While most of the basic command s are similar, there are some small differences in syntax between npm and Yarn:
npm install → yarn install
npm add [package] → yarn add [package]
npm uninstall [package] → yarn remove [package]
npm update → yarn upgrade
npm run [script] → yarn run [script]
Despite the differences in syntax, both accompli the same tasks.
=> Community Adoption and Ecosystem
- npm is the default package manager and has a larger community since it comes bundled with Node.js, so most developers use it by default.
- Yarn gained popularity primarily because of performance improvements and it has since been widely adopted by many organizations. However, as npm has improved its performance and added similar features, Yarn has seen some decline in its dominance.
That said, npm is still the most widely used package manager for Node.js, but Yarn remains a solid choice for teams looking for better performance and specific features like workspaces and deterministic installs.
=> Security
- npm: In the past, npm was criticized for security vulnerabilities, especially with outdated packages. However, npm has since introduced features like audit and audit fix to help identify and resolve security issues in dependencies.
Example command: `npm audit`
- Yarn: Also has built in security features to ensure the integrity of packages.
Example command: `yarn audit`
Both npm and Yarn now provide strong security mechanisms to check for vulnerabilities.
=> Which One to Choose?
Both npm and Yarn are capable of handling Node.js dependencies efficiently. The choice between them typically boils down to the following factors:
Use Yarn if:
- You need faster dependency installation, especially for larger projects.
- You prefer deterministic builds (i.e., predictable, repeatable installations).
- You are working with a monorepo and need workspace support.
Use npm if:
- You prefer the default, built in package manager for Node.js (npm is bundled with Node.js).
- You want to stick with the most widely adopted tool in the JavaScript ecosystem.
- You are using npm’s latest features like npm 7+ workspaces.
Both tools for NodeJS are interchangeable in terms of functionality, and you can switch between them relatively easily. However, you could use either npm or Yarn consistently throughout a project to avoid conflicts, especially with lock files.
=> Example of Switching Between npm and Yarn
If you want to switch between npm and Yarn, make sure to delete the lock file of the current manager before switching to the other one:
1) Switch from npm to Yarn:
Delete `package lock.json`.
Run `yarn install` to generate a `yarn.lock` file.
2) Switch from Yarn to npm:
Delete `yarn.lock`.
Run `npm install` to generate a `package lock.json` file.
2. Express.js versus Fastify: Frameworks for Building Microservices
Both Express.js and Fastify are popular node.js web frameworks for building microservices in Node.js.
=> Express.js
When to Use:
- If you’re looking to quickly prototype or build simple microservices, Express is a great option due to its minimalistic design.
- If you need a well ecosystem that consists of third party tools, plugins and resources.
=> Fastify
Fastify is actually a new Node.js framework and that is basically designed for high performance and low latency applications. It is optimized for building microservices and APIs.
When to Use:
- If your microservices need to hand le a large number of requests per second, Fastify’s performance is a major advantage. So, it makes high performance
- API Heavy Services: Fastify is good for building REST APIs with built in support for request validation and routing.
3. Jest vs Mocha Testing Framework
=> Jest
- For simpler, faster unit tests that require very little setup, Jest is preferable Ideal for React, Next. js, and for modern JavaScript projects
- parallel execution in Jest
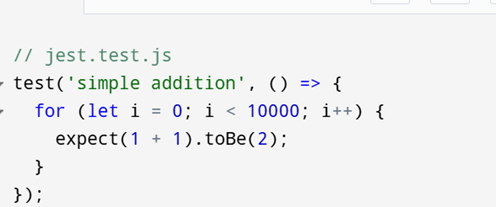
- If you want something fast, all-in-one, and zero configuration, use Jest. It’s great for React, Next. js, modern JavaScript projects.
=> Mocha
- Mocha works well for custom test scenarios, especially in backend applications and microservices, where you need more flexibility.
- Mocha (Sequential Execution)
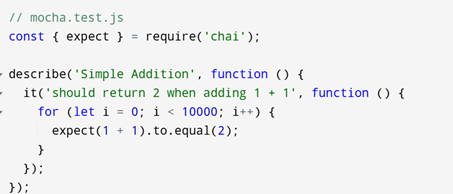
- If you are looking for more flexibility and want to build a customized test setup with Chai, Sinon, and other plugins then you should go for Mocha.
4. RabbitMQ vs. Kafka: Message Brokers for Microservices
Message brokers as you know is really needed in a microservices architecture because it enables asynchronous communication between services. RabbitMQ and Kafka – both are commonly used message brokers and these are suited for different scenarios.
=> RabbitMQ
RabbitMQ supports multiple messaging protocols which includes AMQP.
When to Use:
- Transactional Messaging: If you need guaranteed delivery and message consistency across services should be strong then RabbitMQ’s message queue is ideal in this case.
- High Availability: If your microservices need to be highly available with automatic failover.
=> Kafka
Apache Kafka is a distributed platform for event streaming and it is used to handle high throughput n real time data feeds. Unlike RabbitMQ, Kafka is optimized for large -scale data pipelines and stream processing.
When to Use:
- Event Driven Architecture: Kafka is ideal where you need to build event driven systems where services react to streams of events in real time.
- Analytics in real-time: If you need to process and analyze large streams of any data in real time.
5. Docker | Kubernetes
Containerization (Docker) and orchestration(Kubernetes): Both these are important for deploying your microservices and to manage the deployed svcs in production. Docker and Kubernetes are two main tools for NodeJS which are used for this purpose.
=> Docker
Docker provide platform where it allows you to package your application and its dependencies into a container. And that is why it runs consistently across different environments.
When to Use:
- Deployment Simplification: If you want to easily package your Node.js microservices and deploy them consistently across environments.
- Environment Setup quicker: For local development and testing, Docker provides a simple way to set up microservices and their dependencies.
=> Kubernetes
Kubernetes is orchestration platform and it is open source and widely used where you need to automate the deployment and scaling and management of containerized applications. It is primarily used in conjunction with Docker.
When to Use:
- Microservices in large scale: If you have a complex microservices architecture and need an orchestration platform to manage service scaling, fault tolerance and deployment.
- Multi Cloud or Hybrid Deployments: Kubernetes is best fit for deploying applications across multiple cloud providers or on premises data centers.
6. TypeScript vs. JavaScript: Choice for Microservices
When you are developing microservices in Node.js, to choose between TypeScript and JavaScript is really crucial for long term maintainability, scalability and collaboration.
=> JavaScript
JavaScript is the default language for Node.js development and as I already mentioned, it offers dynamic typing and flexibility.
When to Use:
- Quick Development: If you need to quickly build a prototype or MVP with minimal overhead.
- JavaScript Familiarity: If you are already familiar with JavaScript and prefers the dynamic nature of the language.
=> TypeScript
TypeScript actually a superset of JavaScript and it adds static typing, classes and interfaces. So it helps you to build robust and scalable applications.
When to Use:
- Large Scale Projects: If you’re building complex and large scale microservices that require maintainability and type safety.
- Team Collaboration: If your team values structure and consistent coding practices.
Use Cases and Best Practices of Tools for NodeJS
I have mentioned here about some practical applications of popular tools for NodeJS with complex example implementations to explain how they can be leveraged to solve real world problems.
1. Express.js: Building a Scalable REST API
=> Use Case: A REST API for a Real-Time Inventory System
Let’s say you want to build an inventory management system for an e-commerce platform. This app needs to track real-time updates for products, stock levels and orders. Here I have used Express.js that provides a perfect foundation for developing this RESTful API.
=> Implementation:
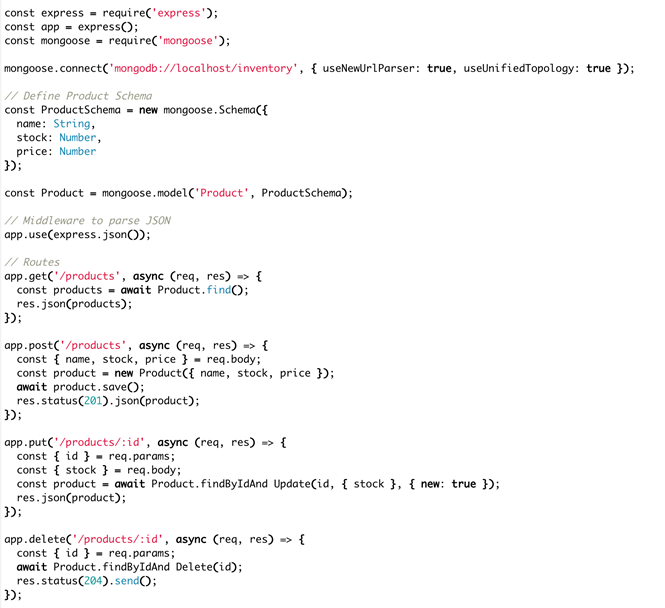
=> Explanation:
- Express.js is used to set up the API server and handle routing.
- MongoDB with Mongoose is used for storing product data in the inventory.
- We define a simple RESTful API with routes for creating, retrieving, updating and deleting products.
You can see here how Express.js can be used to build a scalable and flexible REST API that can managing inventory. You can also include features like authentication, authorization and caching.
2. Kafka: Real-Time Data Processing with Event Driven Architecture
=> Use Case: How to process Real-Time Stock Price Updates
Let’s assume an application that processes real-time stock price updates and then it can send/push notifications if price changes happens. Apache Kafka we can use here as it is distributed event streaming platform and it can handle real-time data feeds.
=> Implementation:
Producer (Stock Price Producer)
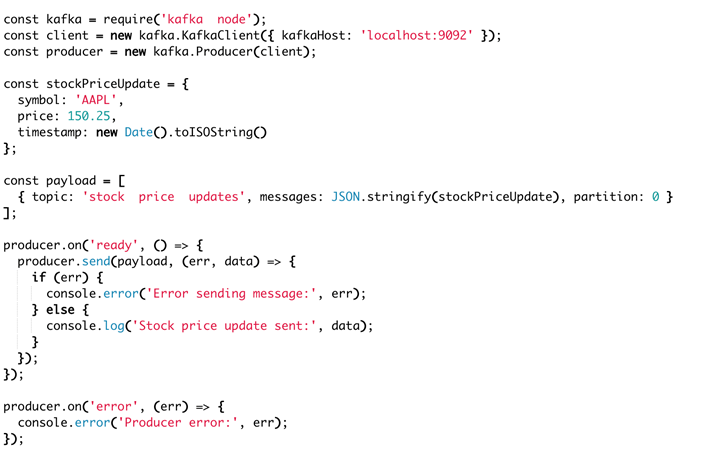
Consumer (Stock Price Consumer)
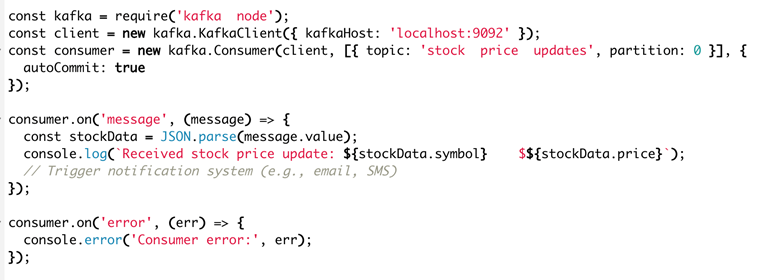
=> Explanation:
- Kafka Producer sends stock price updates as messages to the `stock price updates` topic.
- Kafka Consumer listens to this topic and processes the incoming stock price updates.
- Kafka handles message durability and guarantees delivery, making it an excellent tool for real time event streaming.
3. Docker & Kubernetes: Deploying Microservices in a Containerized Environment
=> Use Case: How to deploy a Microservices based E-Commerce Application
Let’s consider a system that is built with several microservices and app has user-authentication and product catalog and order processing. We can containerize these services using Docker and use Kubernetes to orchestrate and scale them.
=> Implementation:
Step 1: We can Dockerizing the Application
For each microservice, we’ll create a `Dockerfile` to define the environment and dependencies:
Dockerfile
Dockerfile for the User Service
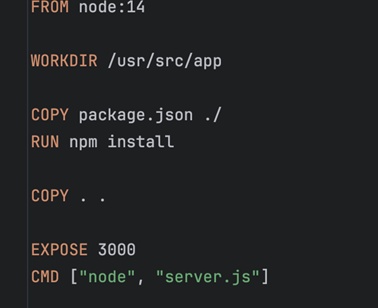
Step 2: Docker Compose for Local Development
docker compose.yml:
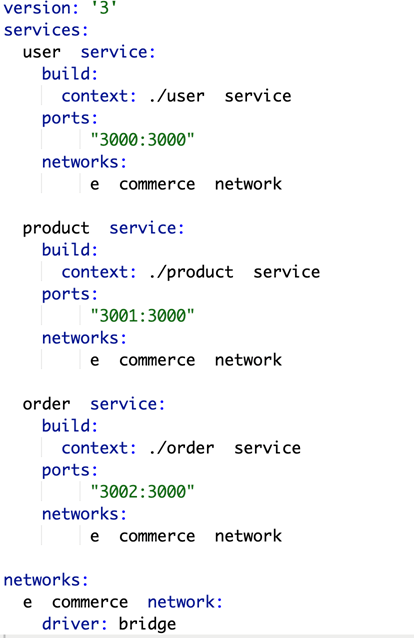
Step 3: Kubernetes Deployment
Once the services are containerized, we can deploy them using Kubernetes. A simple Deployment and Service YAML for one of the microservices (e.g., `user service`) might look like this:
user service deployment.yml:
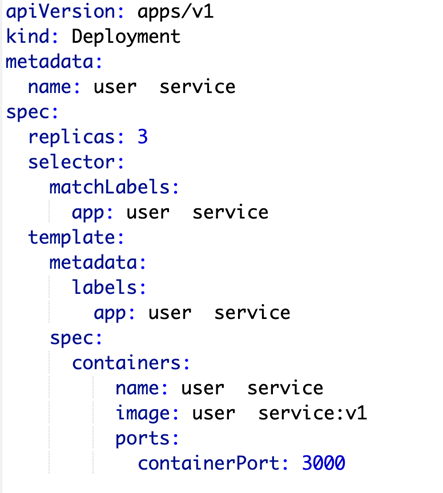
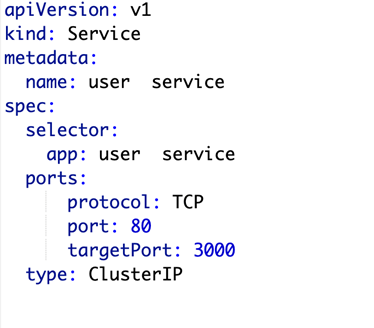
=> Explanation:
- Docker is used to containerize each microservice, ensuring that they can be deployed consistently across different environments.
- Docker Compose simplifies local development by allowing you to define multi container applications with ease.
- Kubernetes automates the deployment, and it scales and manage the containerized services. Also provides features like auto scaling and self healing.
4. TypeScript: Building a Type Safe Microservice
=> Use Case: A Type Safe User Registration Service
Let’s take an simple example where TypeScript improves the development process.
=> Implementation:
user service.ts:
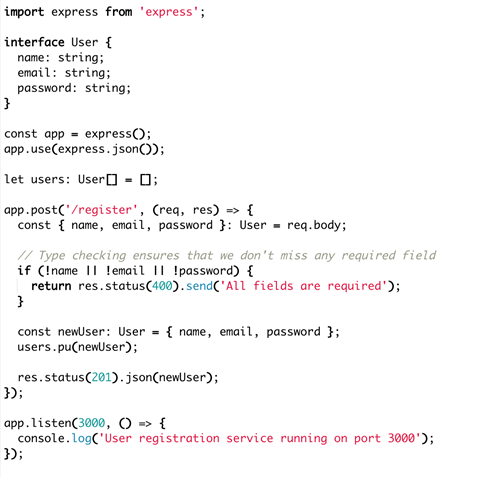
=> Explanation:
- TypeScript introduces static typing to ensure that the `User` interface is strictly adhered to during development and it reduces runtime errors.
- TypeScript compile-time checks guarantee that every necessary field (name, email and password) is present in the request body. It improves reliability and maintainability.
Criteria of selecting Tools for NodeJS
When selecting tools for NodeJS development, consider the following criteria:
1. Express.js: This is ideal for building fast, scalable RESTful APIs. Choose it for simplicity, flexibility and large community support.
2. Kafka: Choose Kafka when you need to hand le high throughput, real time event streaming and ensure reliable message delivery between services.
3. Docker: Select Docker for containerizing applications, ensuring consistency across environments and simplifying deployment processes.
4. Kubernetes: Use Kubernetes for orchestrating and managing containerized microservices, providing auto scaling, self healing and efficient service management.
5. TypeScript: Choose TypeScript for type safety, better code quality and easier debugging, especially in large, complex applications.
Each tool’s selection depends on specific project needs, such as scalability, performance, deployment requirements and the development team’s familiarity.
Best Practices for Seamless Integration and Workflow Optimization in Node.js
Here, I have explained about best practices for seamless integration and workflow optimization in Node.js applications. It ensures smooth development, deployment & maintenance of your complex apps.
1. Modularize Your Codebase with Microservices
=> Why Modularization is Important
We can use Node.js for building microservices based applications due to its lightweight nature and non blocking I/O model.
=> Best Practices
- Decompose/Break pack into Small Services: Each microservice focuses on a specific domain. For example, an e-commerce system can have separate services for users, products and orders.
- Define your Clear API Contracts: Use OpenAPI or GraphQL to define the contracts for APIs between services. This ensures that all services adhere to a standard communication protocol.
- Use REST or gRPC for Communication: RESTful APIs are simple and flexible, but gRPC is a better choice for high performance, low latency communications between microservices.
- Keep your Services to function independently: Make sure each service is independently deployable and can be developed, tested and deployed without affecting other services.
=> Example: Microservice Architecture with Express.js and gRPC
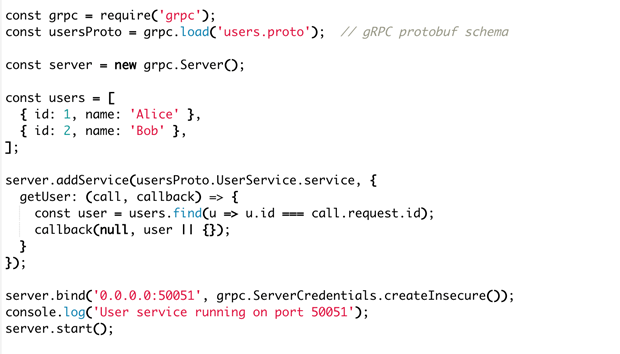
2. Asynchronous Programming for Optimal Performance
Node.js can handle multiple requests concurrently without blocking the event loop.
=> Best Practices
- Use Promises and async/await: Instead of using callback functions, use Promises and async/await to simplify hand ling asynchronous code and avoid callback hell.
- Error Handling: Always handle errors in asynchronous operations using try/catch blocks, `catch()` for Promises, or the `reject()` function in callbacks.
- Async Queues: Use async job queues for managing complex background tasks, such as sending emails or processing images, without blocking the main thread.
=> Example: Asynchronous Task Handling with async/await
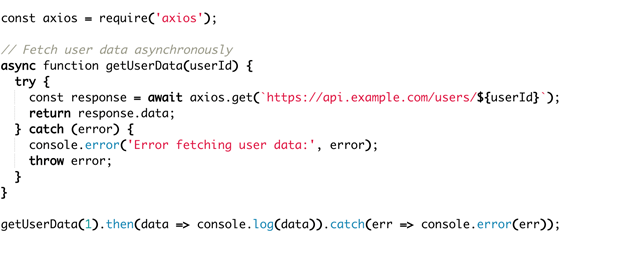
3. Implement Efficient Caching Mechanisms
If you want to reduce the number of requests made to your database or external APIs, then caching can help here and it can improve response times and reduce load on the backend.
=> Best Practices
- Both Redis and Memcached can be used as they are in memory caching solutions and it can cache data at a very low latency. Use of these are ideal for caching frequently accessed data.
- We can cache responses from expensive APIs to prevent redundant calls and can improve user experience.
- Cache expiration policies you can setup and that will make sure caches are properly invalidated when data changes.
=> Example: Caching API Responses with Redis
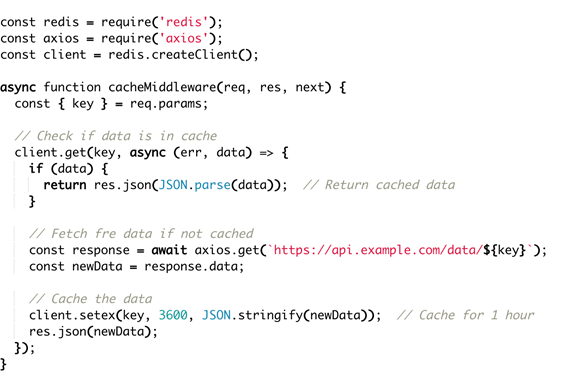
4. Use API Gateway for Simplified Service Management
In Node.js, API Gateway can act as a single entry point for all client requests so that it can simplify routing, load balancing and security.
=> Best Practices
- The API Gateway can handle authentication and authorization centrally, reducing the burden on individual microservices.
- Rate limiting you can implement in the API Gateway that will protect backend services from overloading and ensure fair usage.
- You can use the API Gateway to log and monitor all incoming requests. Also it will provide valuable insights into application performance and health.
=> Example: Using Kong API Gateway
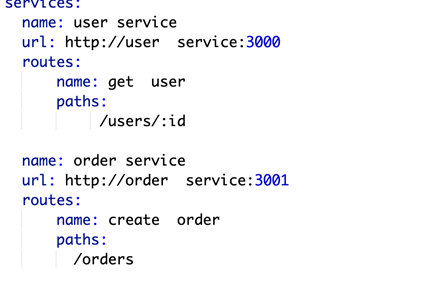
5. Monitoring and Observability with Distributed Tracing
In a microservices, real challenging task is to tracking and monitoring requests across multiple services. Distributed tracing tools such as OpenTelemetry or Jaeger can help track requests through different services.
=> Best Practices
- You can leverage tools like Open Telemetry that can trace requests across diff svcs, so that you can visualize the path of a request and pinpoint performance issues.
- ELK Stack (Elasticsearch, Logsta and Kibana) or Prometheus you can use for logging and metrics aggregation, so that you can gain insights into your system’s health.
- Automated alerts also you can configure based on specific thresholds for application errors, latency, or downtime.
=> Example: Implementing OpenTelemetry in Node.js
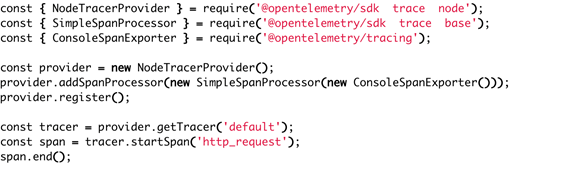
6. Automate Testing and Continuous Integration (CI/CD)
Automated testing can be integrated in your CI/CD pipeline and can verify that changes are thoroughly tested before deployment and it can reduce the risk of introducing bugs into production.
=> Best Practices
- You can use Jest or Mocha frameworks for unit testing individual functions and components of your Node.js application.
- You can use Cypress or Selenium for automated browser testing of your application’s workflows.
- Set up your CI/CD pipelines using tools for NodeJS like Jenkins, GitLab CI, or GitHub Actions so that it will automatically run tests and deploy code.
=> Example: Jest Unit Test for Node.js
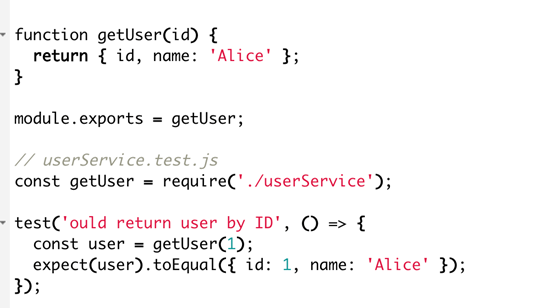
7. Security with Authentication and Authorization
=> Best Practices
- JSON Web Tokens (JWT): It can be used for stateless authentication. It will enable secure communication between microservices.
- OAuth2 for 3rd party Authentication: Those applications that integrate with third party services, in that case OAuth2 you can use to authorize access securely.
- RBAC (Role Based Access Control): You can implement Role Based Access Control to restrict access to certain services or actions based on user roles.
=> Example: JWT Authentication in Express.js
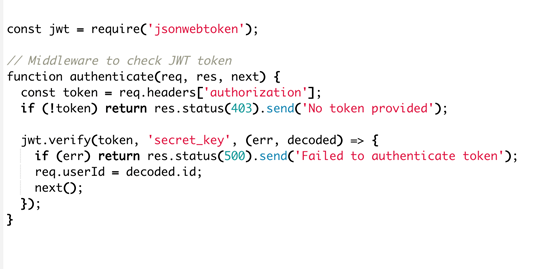
Popular AI-Powered Tools for NodeJS
Here, I have explained about AI-powered tools for NodeJS that are available in current market for NodeJS developers to streamline their workflow and optimize their applications. Let’s explore!
1. The Role of AI in Code Optimization and Debugging
=> Why Use AI for Optimization and Debugging?
- AI powered tools for NodeJS scan your code and runtime performance using custom machine learning (ML) models, natural language processing (NLP) and predictive analytics. They detect potential bottlenecks, bugs, security vulnerabilities and areas for optimization that manual inspection would overlook.
- They can automatically recommend optimizations based on patterns found in large codebases which minimize development cycles, increases code quality and boost performance
=> Key Benefits
- Automated Code Review: AI based systems can scan code for possible problems and propose fixes on their own.
- Performance Tuning: AI can find performance problems by tracking code executions and optimize the performance parameters.
- Bug Detection: Genuinely intelligent debugging systems can foresee problems and fix them or propose means to mitigate them.
- Reduced Development Time: With automated code analyzes and automated fixes, a software developer is more of a feature builder than a bug or performance issue tracker.
2. AI-Powered Tools for NodeJS Code Optimization
A) Sourcery (AI-based Code Refactoring)
Sourcery is an AI-driven code refactoring tool that eliminates redundancies and improving performance. Although it’s more commonly associated with Python, Sourcery’s concepts can also be applied to JavaScript through its advanced code analysis capabilities.
=> Features
- Code Quality Assessment: Sourcery reviews your code and makes recommendations based on how easy your code is to read and how well it performs.
- Automated Refactorings: It enhances the design of existing code by making it more efficient while keeping the behavior the same.
- Performance Improvements: By AI, it recommends changes to improve how much memory is used and how fast the program runs.
=> Example Use Case
- Automatically transforming inefficient recursive functions into iterative ones to reduce stack overflow risks in large scale Node.js applications.
B) DeepCode (AI Powered Code Review)
DeepCode uses AI and machine learning to perform in depth static code analysis. It provides intelligent code reviews that go beyond simple syntax checking, offering context aware suggestions based on best practices and historical data.
=> Features
- Real time Feedback: DeepCode links with your GitHub or GitLab repositories and gives feedback in real time for each commit.
- Context Aware Suggestions: In contrast to common linters, DeepCode proposes solutions based on how the code works, aiming to enhance optimization and readability as well as performance.
- Security Vulnerabilities: DeepCode can detect also security problems such as SQL injection attacks, cross site scripting (XSS), and other security holes.
=> Example Use Case
Detecting a potential security vulnerability like improper input validation that could lead to an XSS attack in your Node.js application.
C) CodeGuru (AWS AI-Powered Code Reviews)
Amazon CodeGuru is an AI-powered tool that provides automated code reviews for Java and Python applications. It uses machine learning models trained on thousands of Java codebases to provide context sensitive feedback.
=> Features
- Code Reviews: CodeGuru automates the process of code review, It identifies issues like memory leaks, resource management and concurrency issues.
- Performance Recommendations: It suggests performance optimizations, especially for memory usage and CPU intensive operations.
=> Example Use Case
Identifying inefficient use of asynchronous operations that may lead to high memory consumption in a Node.js application deployed on AWS Lambda.
3. AI-Powered Tools for NodeJS Debugging
A) Rookout (Intelligent Debugging)
Rookout is AI-powered tools for NodeJS debugging that provides a real-time debugging experience, enabling teams to understand what’s happening in production, find issues and fix bugs more quickly.
=> Features
- Live Debugging: Using Rookout you can set breakpoints on live prod code where you don’t need to redeploy or stop services.
- AI Powered Insights: It uses AI where it can suggest potential areas of the code that may cause issues such as memory leaks, slow database queries or any other performance bottlenecks.
- Context Aware Debugging: Rookout analyzes the code context and offers suggestions for resolving specific issues in the context of the application’s flow.
=> Example Use Case
Debugging intermittent memory leaks in a Node.js application running in a serverless environment.
B) Sentry (AI-Powered Error Tracking)
Sentry is an open source error tracking tool that can be integrated seamlessly with Node.js applications and provides automated AI-driven error tracking and performance monitoring.
=> Features
- Error Aggregation: Sentry uses machine learning to group similar errors, reducing noise and making it easier to pinpoint the root cause.
- Real Time Error Reporting: It automatically captures runtime errors and sends alerts to developers, including detailed stack traces and context around the error.
- Performance Monitoring: Sentry also offers performance monitoring to help developers identify slow endpoints and optimize them.
=> Example Use Case
Identifying an uncaught exception in a Node.js service, pinpointing the exact line in the code that caused it and offering suggestions for better error hand ling.
C) Bugfender (AI Powered Logging and Debugging)
Bugfender is a cloud based tool designed to log and monitor issues in mobile and web applications, and can be used for real-time logging and debugging.
=> Features
- Remote Debugging: Using Bugfender you can view logs and debug applications remotely without needing access to the server or the device.
- AI-Powered Log Analysis: Bugfender uses AI to analyze log data and provide insights into application issues for example: performance bottlenecks or unusual behavior patterns.
- Cra Reports: It offers cra reporting tools for NodeJS to help track and resolve issues that cause application craes.
=> Example Use Case
Debugging performance degradation in a Node.js application by identifying spikes in specific error logs over a period of time.
Integration of Node.js with Modern Cloud Native Architectures
Here I have explained how Node.js integrates seamlessly with cloud native architectures.
1. Understanding Cloud Native Architectures
=> What is Cloud Native?
A cloud-native architecture is a set of recommendations which helps for the design and development of applications and this leverage the cloud to its full potential. Cloud-native applications are built basically by adhering to the following principles:
- Microservices
- Containerization
- Orchestration
- DevOps and CI/CD
- Immutable Infrastructure
2. Why Choose Node.js for Cloud Native Applications?
Node.js is best fit for cloud native applications as it is non-blocking in nature and it has event driven architecture and lightweight footprint.
Why Node.js is a popular choice for building cloud native applications:
- Asynchronous and Event Driven: Node.js can manage asynchronous Input/Output and it very suitable for high-level concurrent applications such as APIs, messaging, and real-time data handling.
- Lightweight and Fast: Compared to conventional applications hosted on servers, Node.js applications actually are faster and easy to deploy.
- Scalability: Node.js can support thousands of concurrent users at the same time.
If we integrate Node.js with cloud native architecture then the result will be a scalable applications we can build that are highly resilient and optimized for the cloud.
In this example, I have explained about integration of Node.js with a cloud native application. This application will showcase the integration of Node.js with Kubernetes, Docker, Microservices, Service Me and CI/CD pipelines. The system will be a simple e-commerce platform with various services like Product Service, Order Service and User Authentication Service.
Scenario Overview
We are building a cloud native e-commerce application with the following features:
- Product Service: Manages products available for purchase.
- Order Service: Manages customer orders.
- User Authentication Service: Hand les user authentication and authorization.
Each of these services will be a Node.js based microservice, deployed in Docker containers and managed using Kubernetes. We’ll also integrate with a service me (like Istio) to manage service to service communication securely.
1. Microservices Architecture
The architecture will consist of three separate Node.js microservices:
- Product Service: A Node.js service providing REST APIs for retrieving product details.
- Order Service: A Node.js service that manages order placement and retrieves product data via the Product Service.
- User Authentication Service: A Node.js service that authenticates and manages users via JWT (JSON Web Tokens).
Each of these services will interact via REST APIs and they will communicate with each other through HTTP requests. They will be orchestrated and scaled using Kubernetes.
2. Node.js Microservices Dockerization
Let’s first create Docker containers for each of our services. We’ll write a `Dockerfile` for each service.
Product Service (Node.js)
Dockerfile for Product Service
- Use official Node.js image from Docker Hub
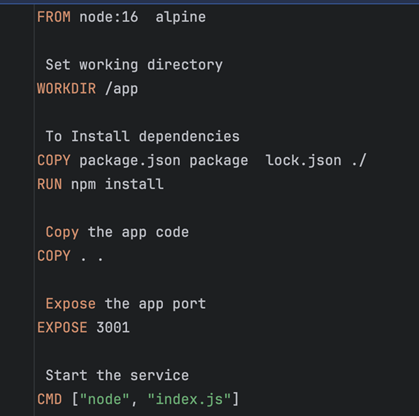
Order Service (Node.js)
Dockerfile for Order Service
- Use official Node.js image from Docker Hub
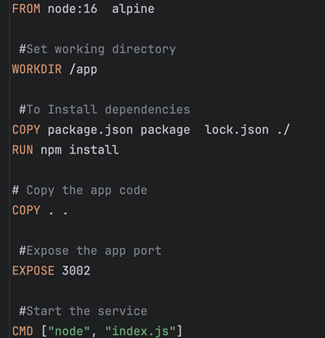
User Authentication Service (Node.js)
Dockerfile for Authentication Service
- Use official Node.js image from Docker Hub
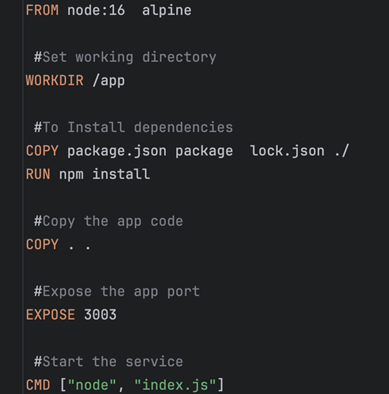
docker compose.yml for Local Testing (Optional)
For local development, we can use Docker Compose to run all services in separate containers on a single host.
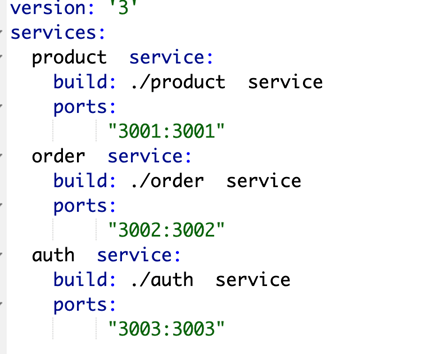
This allows you to quickly spin up all the services locally.
3. Deploying on Kubernetes
Once the services are containerized, we can deploy them on Kubernetes using Deployment and Service resources. Kubernetes will automatically handle scaling, failover and load balancing.
=> Kubernetes Deployment for Product Service
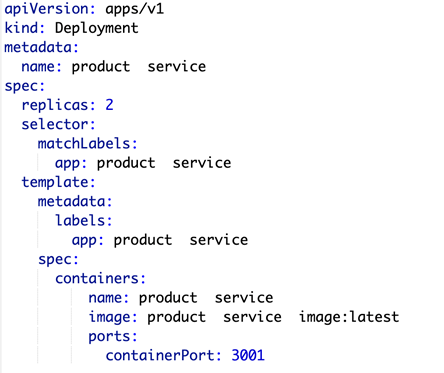
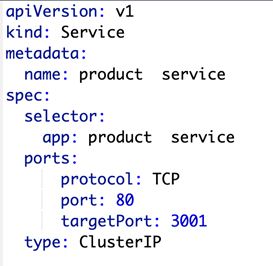
=> Kubernetes Deployment for Order Service
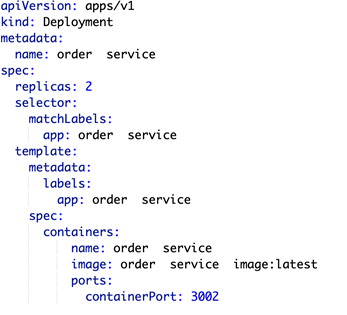
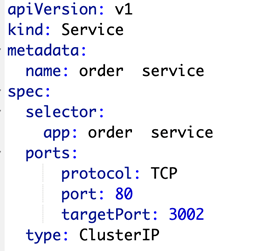
=> Kubernetes Deployment for Authentication Service
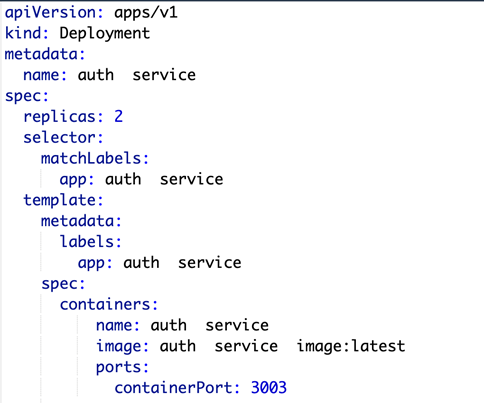
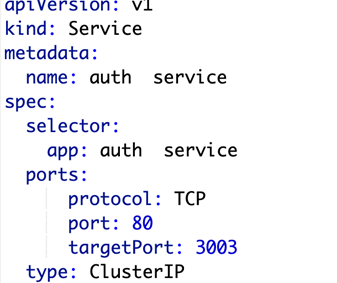
=> Service Me with Istio
First, we’ll deploy Istio and configure the services to communicate with each other securely via Istio’s sidecar proxy.
Install Istio:
- istioctl install set profile=demo
Enable automatic sidecar injection for your Kubernetes namespaces:
- kubectl label namespace default istio injection=enabled
Istio Gateway for External Access
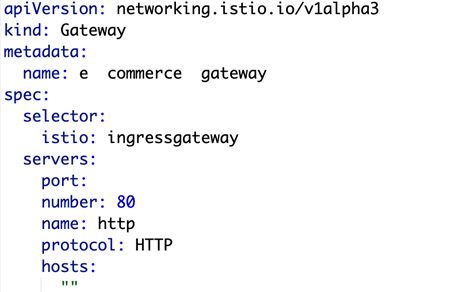
4. CI/CD Pipeline with Jenkins
We’ll automate the build, test and deployment process using Jenkins. Below is a simple Jenkinsfile for deploying the Node.js services to Kubernetes.
=> Jenkinsfile Example:
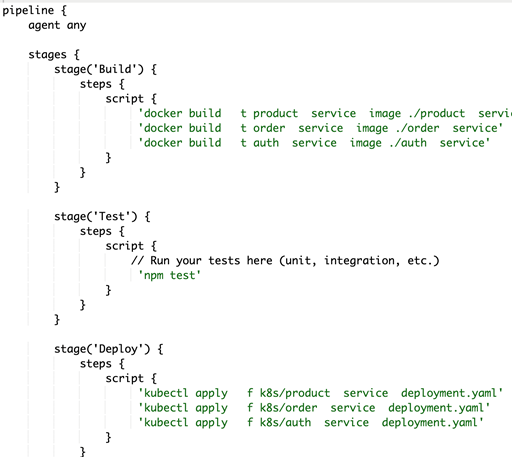
=> This Jenkins pipeline automates
- Docker image build for each service.
- Running tests (you can add test scripts in each service).
- Deploying the services to a Kubernetes cluster.
- Once deployed, use Kubernetes Horizontal Pod Autoscaler (HPA) to automatically scale the microservices based on CPU or memory usage. You can also integrate Prometheus and Grafana for monitoring, ensuring the performance of each service.
Conclusion
=> How important it is to select the right tools for NodeJS development
All the tools for NodeJS development helps enhance efficiency, maintainability, and performance. Developing the ideal mix of editors, testing frameworks, automation systems, and debugging tools for NodeJS will help the developer produce efficient working programs. While ensuring fewer bugs come into the system, thereby increasing production efficiency.
Whereas Jest is great for test and easy test with less configuration, Mocha is versatile and fits in complex workflows. Like with all tools for NodeJS, one’s choice of VS Code, WebStorm, or Sublime simply comes down to personal preference and what the project requires.
=> How important it is to do experience and prioritize workflow customization
Improving productivity is one of the phrases developers think about, however attaining that is difficult. Developers are continually considering strategies that can be adopted to boost productivity. Simple tweaks like using new tools for NodeJS, installing different plugins, or even altering the configurations can improve workflow to a great extent.
Although customization can be complex, integrating tools for NodeJS to suit the specification of an development project improves productivity and experience. Lastly, remaining flexible and using proper tools for NodeJS enables quicker development and higher quality code in the continuously adjusting environment.
Read more: