What is Firebase Authentication in Node.js?
Firebase Authentication in Node.js is basically a secure user authentication system and it is developed by Google designed to simplify the sign-in process for web and mobile applications. It provides authentication mechanisms for user identity management and it supports various sign-in methods including:
- Email & Password Authentication – This is traditional login using credentials.
- Social Authentication – Where you need to sign in with Google, Facebook, Twitter or GitHub.
- Phone Authentication – OTP-based authentication.
- Anonymous Authentication – Temporary access for guest users.
- Custom Authentication – Using JWT tokens for advanced security needs.
By integrating Firebase Authentication in Node.js applications, the Node.js development services provider can eliminate the complexity of managing user sessions. Also, can secure passwords and implement Multi-Factor Authentication (MFA).
Why do you need Firebase Authentication in Node.js Applications?
Security and Compliance
- Firebase Authentication in Node.js gives a secure authentication framework which follows industry-standard protocols such as OAuth 2.0 | OpenID Connect | JWT-based authentication. This helps in preventing security threats such as unauthorized access and identity fraud.
Simplified User Management
- One thing is that to manage user authentication and to handle session in traditional authentication systems is really complex. Firebase Authentication simplifies this as it offers pre-built UI flows | SDKs and APIs that seamlessly integrate into Node.js applications.
Scalable and Cost-Effective
- Node.js applications often require a backend that supports millions of users. Firebase Authentication is cloud-based and scalable it means that it grows with your application’s demand and it does not require manual infrastructure management.
Seamless Integration with Firebase Ecosystem
- Firebase Authentication works natively with Firebase services like Firestore, Cloud Functions, and Real-Time Data Streaming with Node.js. It enables and simplify the Node.js architecture.
Multi-Platform Compatibility
- It allow Nodejs developers to use the same authentication logic across web, mobile (iOS/Android) and backend (Node.js) applications so that it ensures consistent user experiences.
Advantages of using Firebase Authentication
- Easy Integration: It makes user authentication simple and without complex backend implementation.
- Secured: It uses industry-standard security practices like OAuth 2.0 and OpenID Connect.
- Scalable: Also it handles thousands of users without manual infrastructure scaling.
- Cross-Platform Support: It works seamlessly with web mobile & backend applications.
Real-Time Use Cases of Firebase Authentication
- E-Commerce Platforms: Ensures secure user logins for shoppers and enables social authentication for quick sign-ups.
- SaaS Applications: Allows users to sign up and authenticate with Google, Facebook or GitHub. Manages multi-user access with roles and permissions.
- IoT Applications: Secures API endpoints and authenticates device access.
- Online Learning Platforms: Manages student and instructor logins securely.
How to set up Firebase Authentication in Node.js Application
Step 1: Create a Firebase Project
=> Go to Console of Firebase.

=> Click on “Add Project” and set up a new Firebase project.
=> Enable Authentication: Navigate to Authentication > Sign-in Method, then enable Email/Password and other sign-in providers.
Step 2: Install Firebase Admin SDK
=> npm install firebase-admin
Step 3: Initialize Firebase Authentication
=> Create a firebase.js file to set up Firebase Authentication:
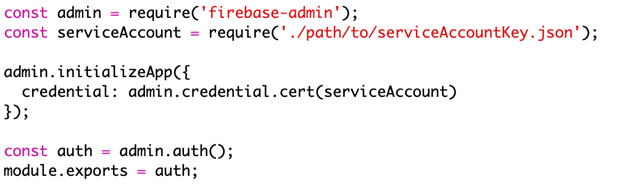
NOTE: Download ‘serviceAccountKey.json’ from Firebase Console under Project Settings > Service Accounts.
Step 4: User Registration (Sign-Up)
=> Create a `registerUser` function in authController.js:
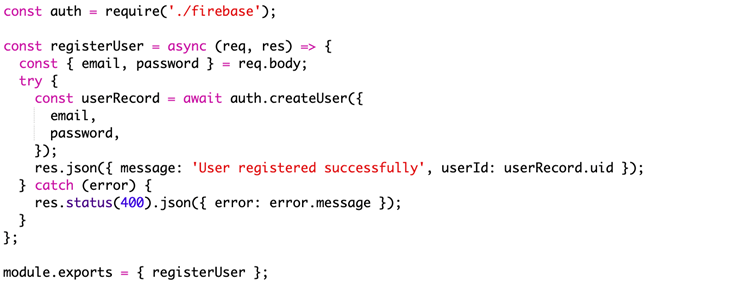
Step 5: User Login with Firebase Authentication
=> To allow users to log in, use Firebase Client SDK on the frontend.
Example React Login Component:
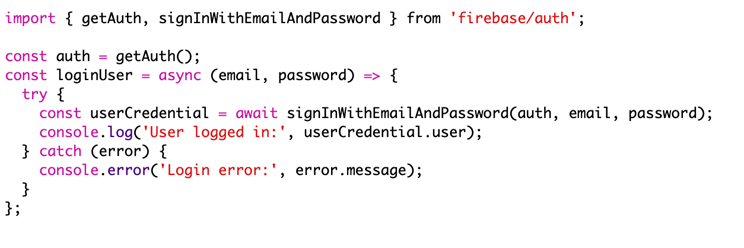
Step 6: Protecting API Routes (JWT Authentication)
=> To protect API routes, use Firebase’s `verifyIdToken` function.
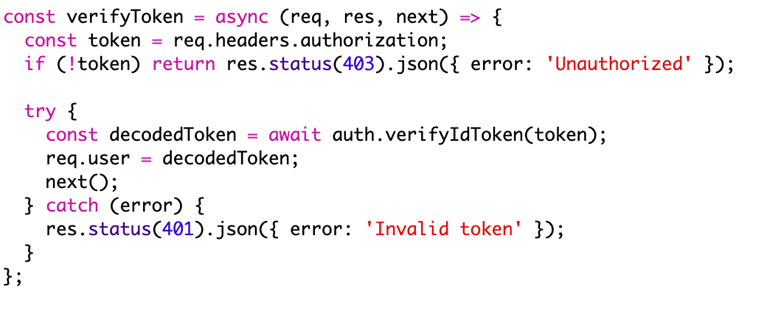
=> Use this middleware in protected routes:

Example: Implementation of Firebase Authentication in Node.js based Microservices
Firebase Authentication as I already mentioned it simplifies user authentication in Node.js as it provides a secure and scalable identity management solution. In this blog, I have explained the implementation of Firebase Authentication in Node.js based microservices framework using a real-time microservice application example.
Key Components
- Authentication Service: Handles Firebase authentication, user login, and registration.
- User Service: Manages user profile information.
- Order Service: Processes customer orders.
- Product Service: Manages product inventory and listings.
- API Gateway: Handles authentication and routes requests to respective microservices.
Tech Stack Used
- Node.js + Express.js/NestJS for building microservices.
- Firebase Authentication (Admin SDK) for secure user authentication.
- Kafka/RabbitMQ for event-driven communication between microservices.
- Redis for caching authentication tokens.
- Docker + Kubernetes for containerization and scaling.
Step-by-Step Implementation
Setting Up Firebase Authentication
- Install Firebase Admin SDK
- npm install firebase-admin express dotenv
Initialize Firebase Admin in `authService.js`
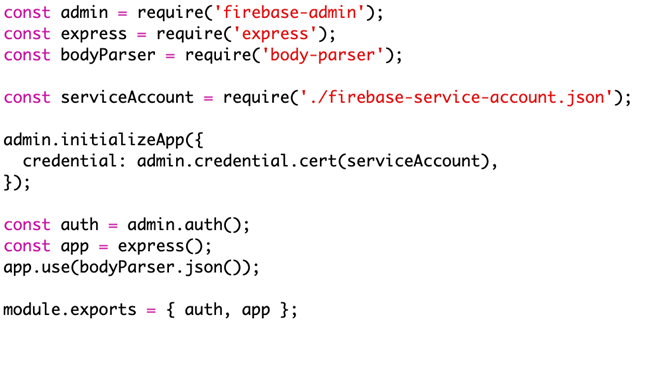
Implementing User Registration & Login APIs
User Registration API
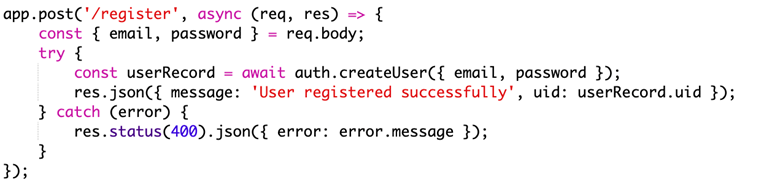
User Login & Token Verification
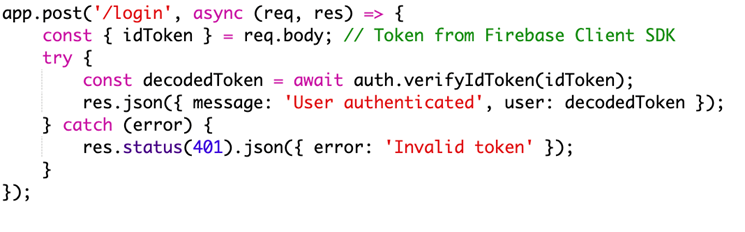
How to Secure your Microservices with Firebase Authentication
To protect API routes, we will use a middleware function to verify Firebase tokens before allowing access to microservices.
=> Authentication Middleware (`authMiddleware.js`)
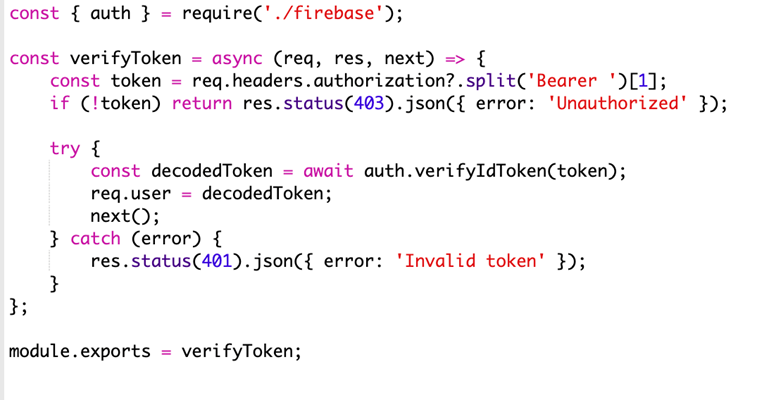
=> Protecting Routes in Microservices (e.g., Order Service)
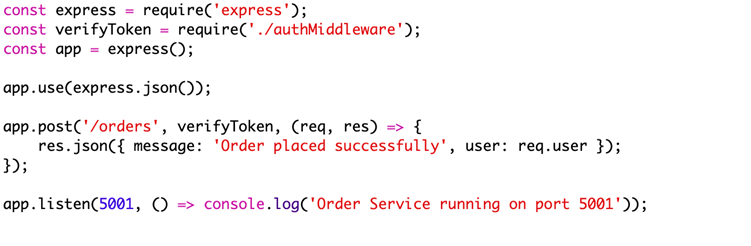
=> API Gateway for Authentication & Routing
The API Gateway will act as an entry point to all microservices, validating authentication before forwarding requests.
API Gateway (`apiGateway.js`)
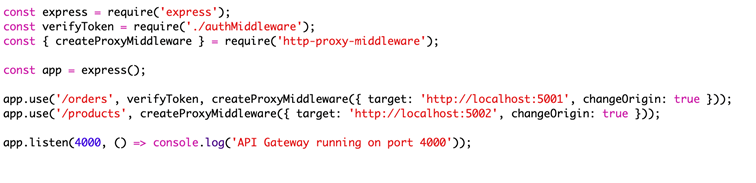
Deploying Node.js Microservices on AWS
=> Containerizing Microservices using Docker.
=> Create a `Dockerfile` for each microservice:
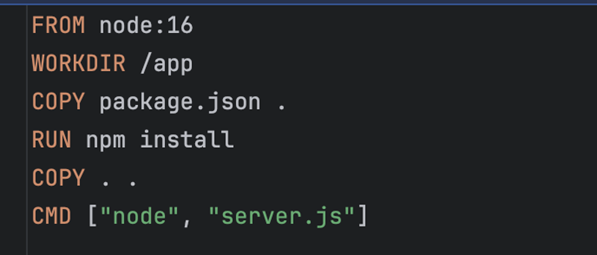
=> Build and push Docker images to Amazon Elastic Container Registry (ECR):



Deploying Microservices on AWS ECS
=> Create a task definition and deploy services using AWS Fargate for serverless scaling.

=> Install and Configure Nginx on EC2
- sudo apt update && sudo apt install nginx -y
=> m ,,k.l
- Configure Nginx as API Gateway (`/etc/nginx/nginx.conf`)
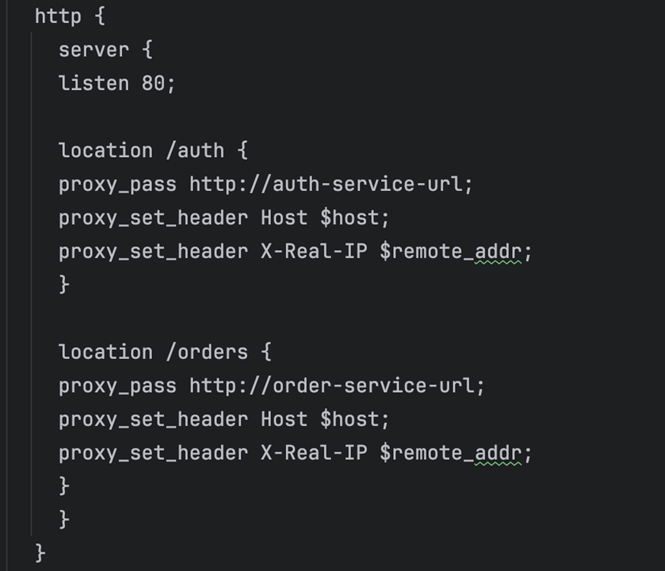
=> Restart Nginx
- sudo systemctl restart nginx
How to Secure API Gateway with Firebase Authentication in Node.js
=> Modify Nginx config to validate Firebase JWT before proxying requests.
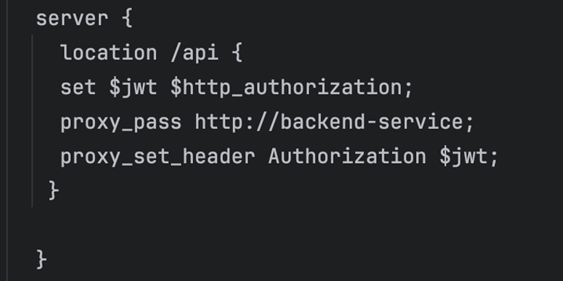
=> Add authentication middleware in microservices.
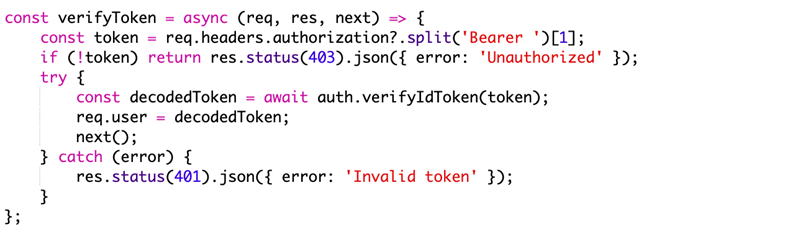
Read more: