Overview of Java Pattern Matching for Switch
Java has continuously improved to provide code-writing tools that are more expressive, legible, and succinct. Pattern Matching for switches is one such improvement; it was first included as a trial feature in Java 17 and became a standard feature in Java 21. Code is clearer and less prone to errors thanks to this feature, which greatly streamlines type checks and casting within a switch construct.
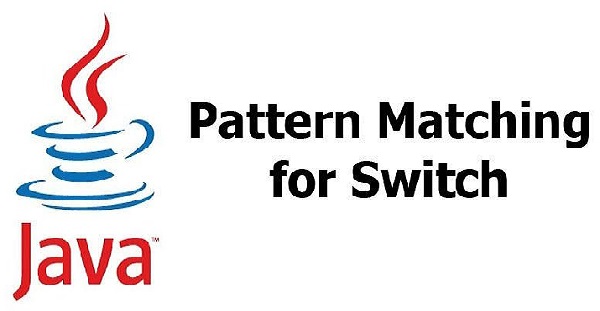
This change aligns with focus on improving the programmer experience of Java Development Company by reducing boilerplate code and increasing readability. By adding support for patterns to the conventional switch statement, Java Pattern Matching for Switch enables writers to:
- Match the type of an object.
- Extract and work with the matched object seamlessly.
- Combine type check and with conditional logic for more expressive control flow.
Key Features of Java Pattern Matching for Switch
- Type Specific Matching: Automatically checks the type of an object and performs safe casting.
- Null Handling: To prevent surprises from NullPointerExceptions, it explicitly permits matching null values.
- Guarded Patterns: For more guarded control it enables type checks with conditions.
- Default Cases: Support fallback behavior for unmatched cases.
READ – Advanced Design Patterns in the Java Collection API
Basic Example of Pattern Matching for Switch
public class PatternMatchingSwitchExample {
public static void main(String[] args) {
Object obj = "Hello, World!";
String result = switch (obj) {
case Integer i -> "Integer: " + (i * 2);
case String s -> "String: " + s.toUpperCase();
case Double d -> "Double: " + (d / 2);
case null -> "Null value";
default -> "Unknown type";
};
System.out.println(result);
}
}
Explanation
- Declaration of variable:
- The ‘obj’ variable is initialized with a string.
- Pattern Matching in Switch:
- Integer i – Matches when an ‘obj’ is an integer and binds the value to ‘i’.
- String s – Matches when an ‘obj’ is a string and binds the value to ‘s’.
- Double d – Matches when an ‘obj’ is a Double and binds the value to ‘d’.
- null – Matches if the object is ‘null’.
- default – Catches all the unmatched cases.
- Result:
- Since ‘obj’ is a string, the case string ‘s’ executes, and s.toUpperCase() converts the string to uppercase.
Output
String: HELLO, WORLD!
Example Using Guarded Patterns
Additional conditions can be included in case statements using guarded patterns. Let’s use a shape hierarchy to investigate this:
public class ShapeExample {
sealed interface Shape permits Circle, Rectangle {}
static final class Circle implements Shape {
double radius;
Circle(double radius) { this.radius = radius; }
}
static final class Rectangle implements Shape {
double length, width;
Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
}
public static void main(String[] args) {
Shape shape = new Circle(5);
String result = switch (shape) {
case Circle c && c.radius > 0 -> "Circle with radius: " + c.radius;
case Rectangle r && r.length > 0 && r.width > 0 ->
"Rectangle with dimensions: " + r.length + " x " + r.width;
default -> "Invalid shape";
};
System.out.println(result);
}
}
Explanation
- Sealed Classes: The ‘Shape’ interface is sealed, and permits only rectangle and circle subclass.
- Guarded Patterns:
- In case Circle c && c.radius > 0 – Matches circle objects with a positive radius.
- In case Rectangle r && r.length > 0 && r.width > 0 – Matches Rectangle object with positive dimensions.
- Default Case: Handle unmatched or invalid shapes.
Output
Circle with radius: 5.0
Advantages of Java Pattern Matching for Switch
The Java Pattern Matching for Switch offers several advantages that simplify and enhance code. It allows for more expressive control flow by enabling conditional logic within the switch cases. This is especially useful when working with complex types.
1) Improve Readability
Removes explicit type checks and casts, which reduces verbosity.
2) Seamless Integration
Works naturally with other features like Sealed Classes in Java.
3) Safer Code
Avoids errors associated with unchecked casts.
4) Declarative Logic
Encourages programming that is more declarative by emphasizing the pattern rather than the details.
Conclusion
Java Pattern Matching for Switch is a strong addition that makes type-safe control flow easier. It assists Java Developers India in writing more expressive and maintainable code by integrating conditional logic, casting, and type checks into a single construct. By leveraging Java Pattern Matching for Switch, development programs become more elegant and maintainable, offering both efficiency and clarity without sacrificing performance.
Read more: