Java 23 & Springboot compatibility:
As of sept 2025, Java 23 has been released officially. On the other hand Spring Boot 3.x is designed such a way that it is compatible with Java versions 17 through 23. This compatibility actually ensures that we can leverage the latest features of Java 23 within their Spring Boot 3.x applications.
Key Compatibility Considerations:
Jakarta EE Migration:
- In Spring Boot 3.x actually it has fully transitioned from Java EE (`javax` packages) to Jakarta EE (`jakarta` packages). This migration basically requires developers to update their imports and dependencies accordingly. For example, ` javax.persistence.Entity` should be replaced with ` jakarta.persistence.Entity`. This change aligns with the broader ecosystem’s shift towards Jakarta EE.
Java 23 Features:
- Java 23 introduces several enhancements and this includes improved pattern matching and the Vector API and generational ZGC. In our Spring Boot 3.x applications, we can utilize these features seamlessly and only criteria is that our application is running on a Java 23 runtime.
Complex Example: Utilizing Java 23 Features in a Spring Boot 3.x Application
Below is an example demonstrating the integration of Java 23’s pattern matching enhancements within a Spring Boot 3.x REST controller:
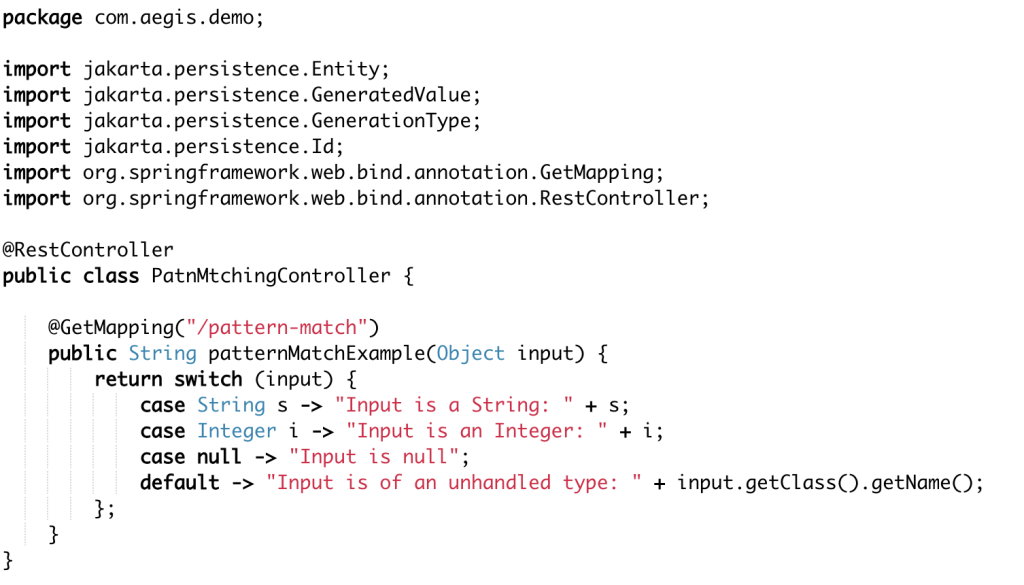
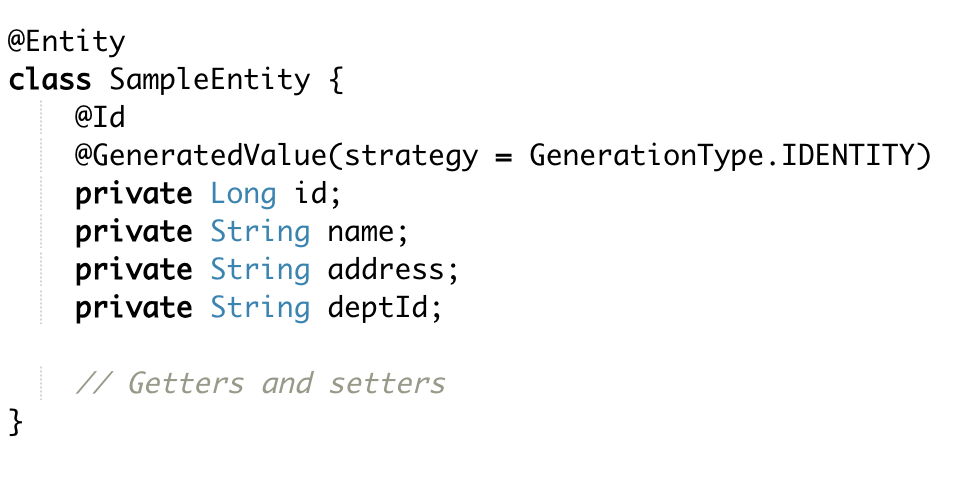
Explanation:
- Pattern Matching in `switch`: The `patternMatchExample` method basically portrays Java 23’s enhanced pattern matching capabilities within a `switch` statement, and this allows for type-specific handling directly in the case labels.
- Jakarta Persistence API: The `SampleEntity` class utilizes `jakarta.persistence` annotations and this basically reflects the necessary migration from `javax.persistence` in Spring Boot 3.x.
Migration Tips:
- Update Dependencies: Ensure all dependencies are compatible with Jakarta EE. This may involve updating library versions or replacing libraries that have not yet migrated to Jakarta EE.
- Refactor Imports: Use tools or IDE features to refactor `javax` imports to `jakarta` equivalents systematically.
- Testing: Thoroughly test the application after migration to identify and resolve any issues arising from the transition.
By adhering to these guidelines, developers can effectively leverage the advancements of Java 23 within their Spring Boot 3.x applications that will definitely ensure modern, efficient, and maintainable codebases.
Integrating Java 23 features into Spring Boot 3.x microservices can significantly enhance development efficiency, performance, and code readability. Below I have explained some key Java 23 features and their implications for Spring Boot microservices in addition with complex use case implementations:
Primitive Types in Pattern Matching and `switch` Statements
Java 23 extends pattern matching to support primitive types in `instanceof` checks and `switch` statements which enables and make it more concise and type-safe code.
Use Case: Dynamic Request Processing Based on Input Type
- In a microservice handling various types of inputs (e.g., JSON, XML, plain text), you can utilize enhanced `switch` statements to process requests dynamically:
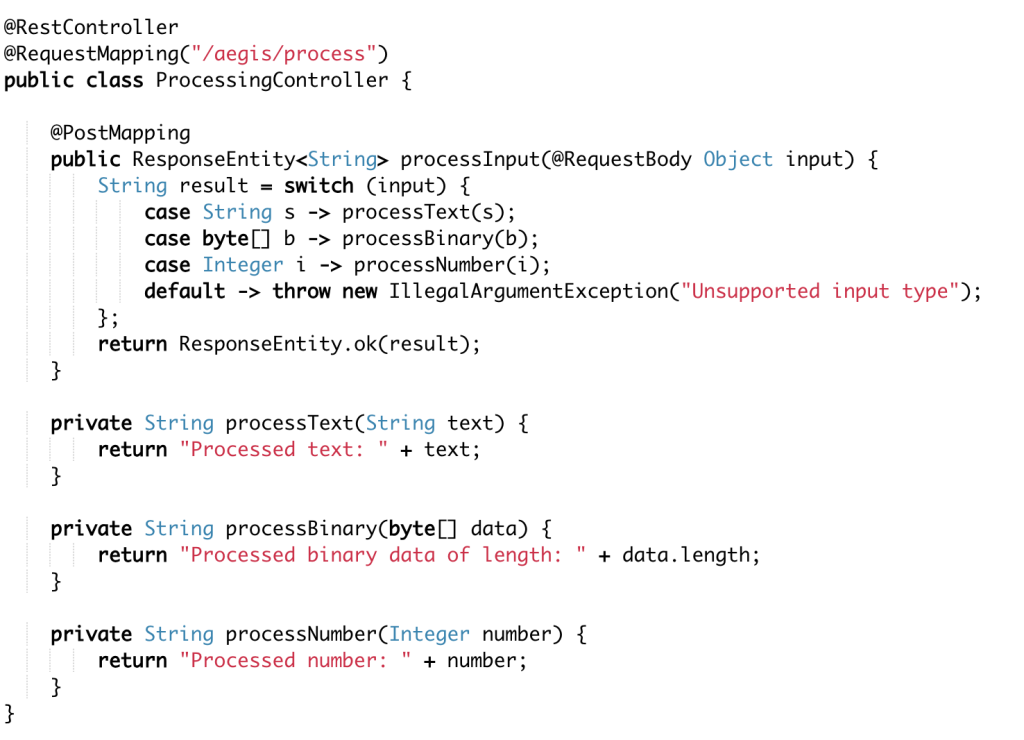
This approach streamlines input handling by leveraging Java 23’s pattern matching capabilities and it basically reduces boilerplate code and enhances maintainability.
Structured Concurrency
Java 23 introduces structured concurrency that basically simplifies managing multiple concurrent tasks by treating them as a single unit of work.
Use Case: Parallel Data Fetching from Multiple Services
- In a scenario what happens actually where a microservice needs to aggregate data from multiple external services and here it may be through various 3rd party API calls and in this scenario, structured concurrency can be employed to perform parallel data fetching:
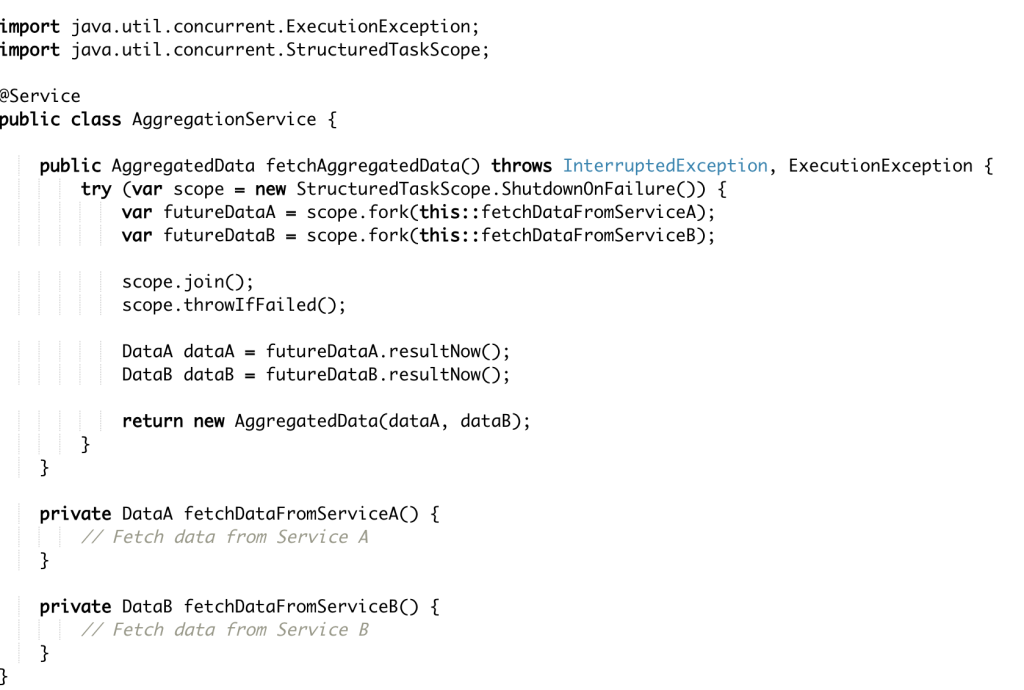
The above implementation ensures that data fetching operations from various svcs are executed concurrently, and this basically improves response times and at the same time it maintains error handling and cancellation policies.
Scoped Values
Scoped values provide a safer and more efficient alternative to thread-local variables, especially useful in microservices handling asynchronous requests.
Use Case: Propagating Context Information in Asynchronous Operations
- When processing requests that require context propagation (e.g., user authentication details) across asynchronous operations, scoped values can be utilized:
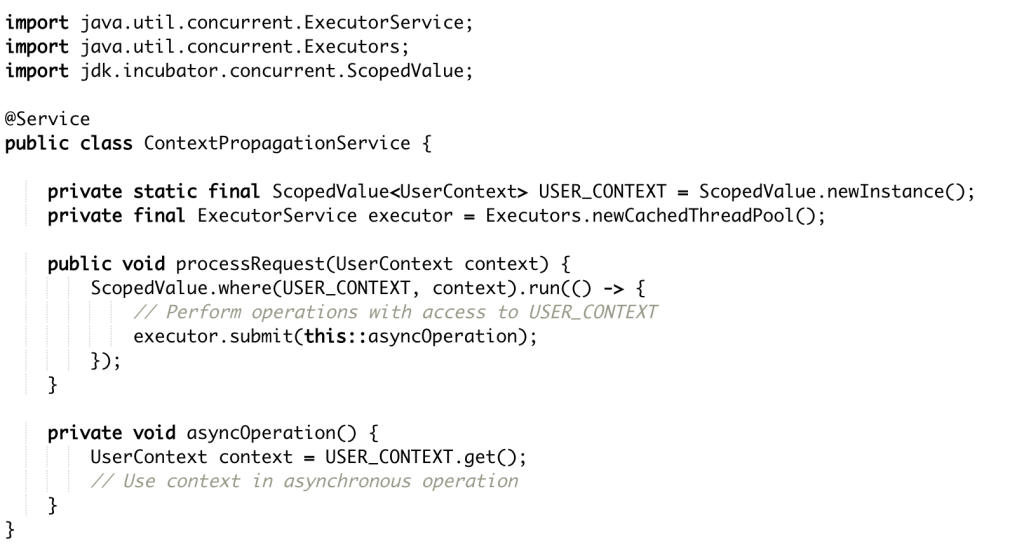
- This pattern ensures that context information is safely and efficiently propagated across asynchronous boundaries without the pitfalls of traditional thread-local storage.
Markdown Documentation Comments
- Java 23 allows the use of Markdown syntax in JavaDoc comments and as a result it basically enhances the readability and maintainability of API documentation.
Use Case: Enhancing API Documentation for Microservices
- In a Spring Boot microservice, we can document REST controllers using Markdown because it provides user-friendly and formatted API documentation:
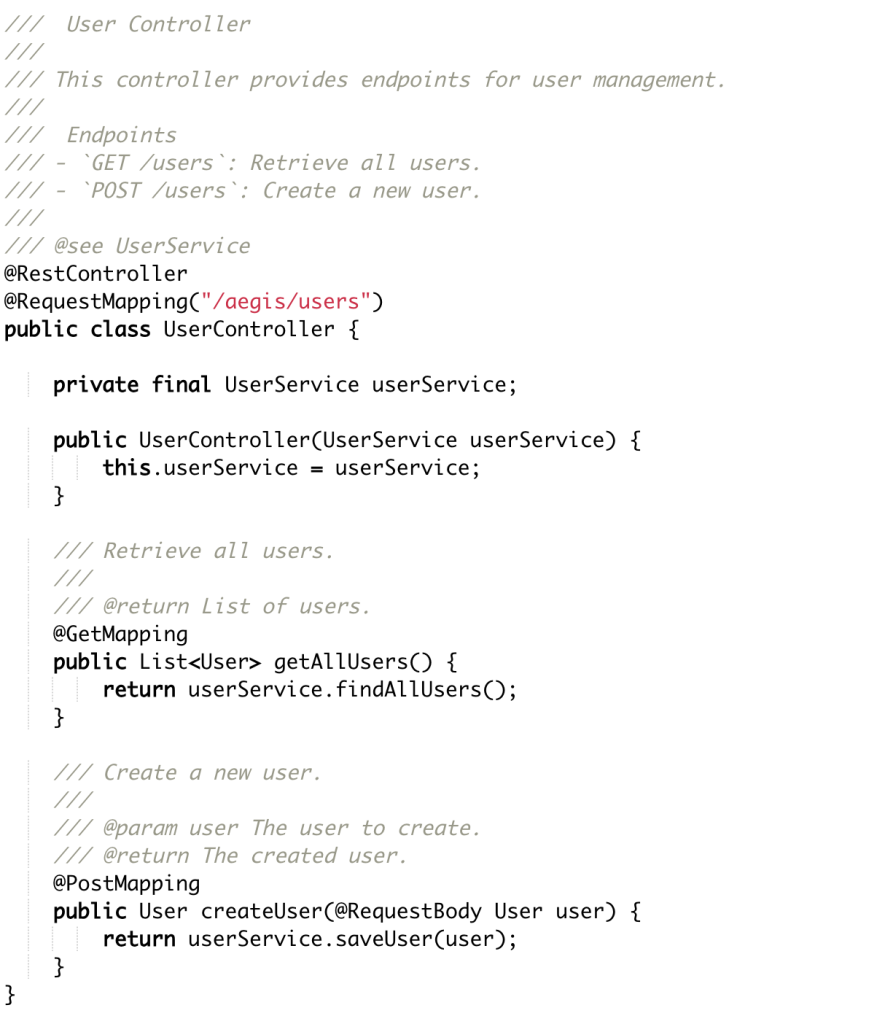
- If we utilizing Markdown in documentation comments, then basically it will improve the clarity and accessibility of API documentation as you can see in above example and this facilitates better collaboration and understanding among development teams.
How to migrate a Spring Boot Microservice from Java 17/21 to Java 23
If you want to migrate your Spring Boot microservice from Java 17/21 to Java 23 and then the major steps would involve updating dependencies, leveraging new java development services features, and ensuring compatibility with Spring Boot 3.x. Below I have explained in-details about the migration with detailed examples.
1. Upgrade Java Version and Dependencies
First, update your project’s JDK version to Java 23.
For Maven:
Modify your `pom.xml`:
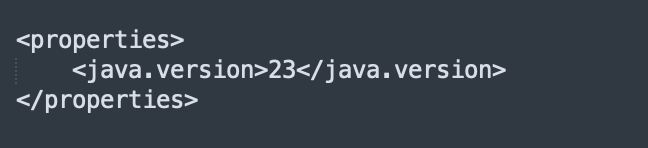
For Gradle:
Modify `build.gradle`: gradle
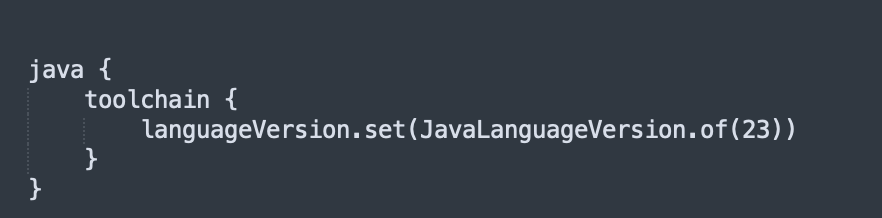
Check Java version:
java -version
Expected output:
openjdk version “23” 2025-XX-XX
2. Update Dependencies
Spring Boot 3.x
Suppose your microservice is currently on Spring Boot 2.x, then you have to update it to latest version of springboot and it is Spring Boot 3.x, because 3.x supports Java 23.
Update `pom.xml`:
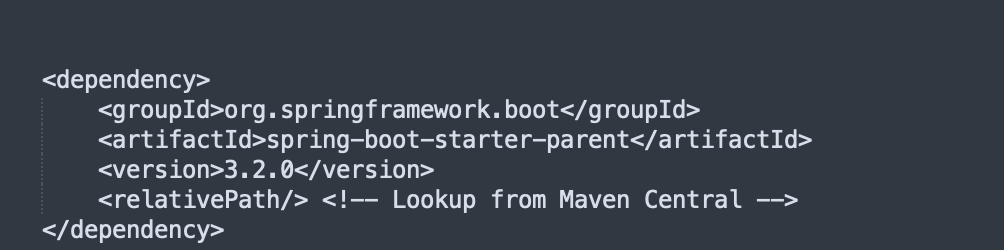
3. Code Changes for Java 23 Compatibility
(A) Refactor `javax` to `jakarta`
Spring Boot 3.x replaces `javax` with `jakarta`. Update imports in your code:
Before (Java 17/21 – Spring Boot 2.x)
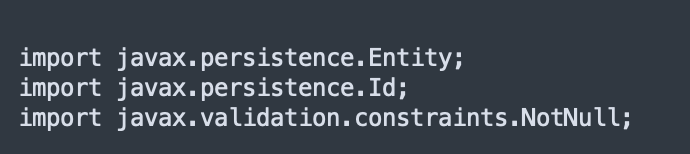
After (Java 23 – Spring Boot 3.x)

If you use `@WebServlet`, `@WebFilter`, or `@WebListener`, update them:

(B) Replace `ThreadLocal` with Scoped Values
Before (Using ThreadLocal in Java 17/21)
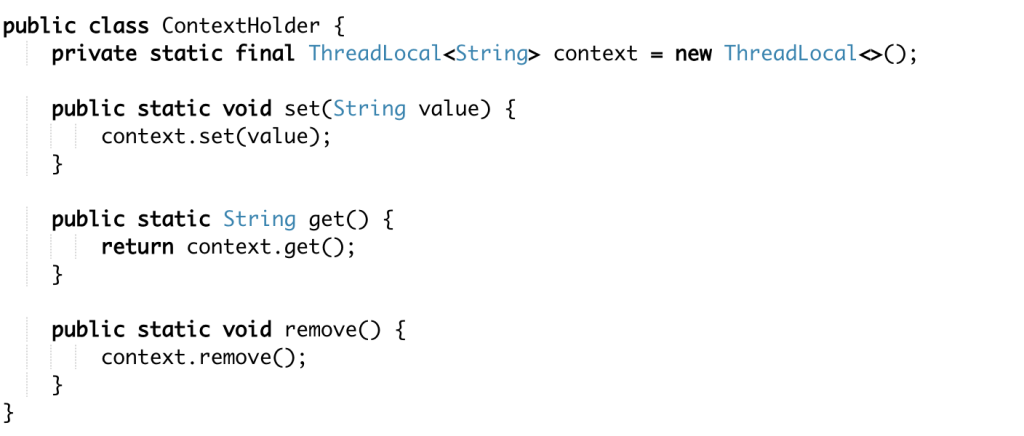
Issue: ThreadLocal is inefficient in reactive Spring applications.
After (Using Scoped Values in Java 23)
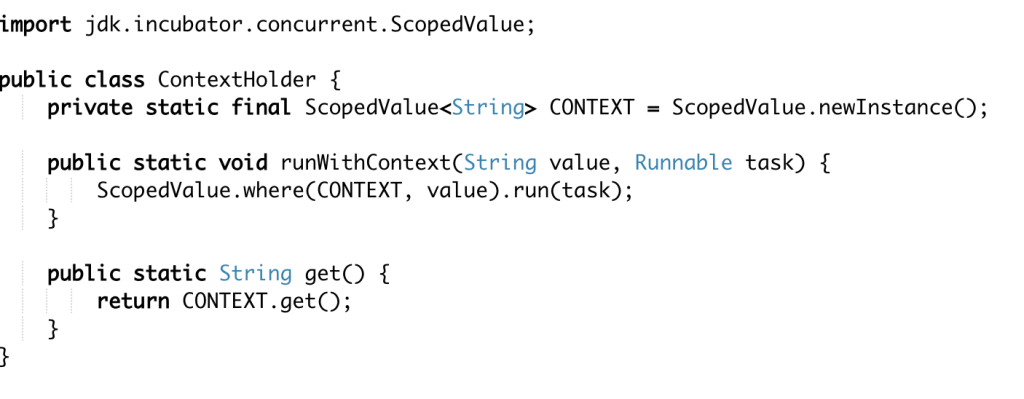
Benefits: Scoped Values are more memory-efficient and safe in asynchronous applications.
(C) Optimize Concurrency with Structured Concurrency
Java 23 introduces structured concurrency, simplifying parallel execution.
Before (Using `CompletableFuture` in Java 17/21)

Issue: Harder to manage errors and cancellation.
After (Using StructuredTaskScope in Java 23)
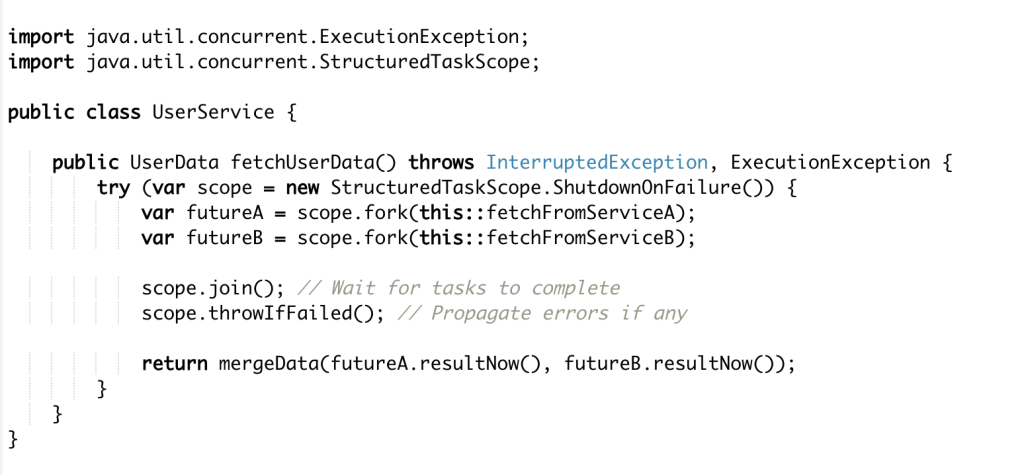
Benefits: Improves cancellation, error handling, and performance.
(D) Use Record Patterns for Cleaner Code
Java 23 extends pattern matching in records.
Before (Java 17/21)
- record User(String name, int age) {}

After (Java 23 – Record Patterns in `switch`)
- record User(String name, int age) {}

Benefits: Improves readability by removing unnecessary type checks.
(E) Use Virtual Threads for Efficient Microservices
Java 23 refines virtual threads, reducing the need for thread pools.

After (Java 23 – Virtual Threads)

Benefits: More lightweight and scalable for microservices.
4. Testing Java 23 Migration
Run the following tests to validate your migration:
Check Java Version
- java -version
Run Unit Tests
- mvn test
Check Jakarta EE Migration
Use Maven Dependency Plugin:
- mvn dependency:tree
You will have to make sure that all your dependencies that you are using should be Jakarta EE compatible.
Conclusion:
Your goal to migrating from Java 17/21 to Java 23 in a Spring Boot microservice is worth because it would improve performance, code readability, and concurrency handling. The key changes include:
✔ Updating `javax` to `jakarta`
✔ Using Scoped Values instead of ThreadLocal
✔ Switching from CompletableFuture to StructuredTaskScope
✔ Utilizing Pattern Matching and Record Patterns
✔ Replacing Thread Pools with Virtual Threads
By following the above mentioned step, your microservices will be optimized for Java 23 as well as it will leverage the latest Spring Boot 3.x capabilities.
Read More: